方式一
1.依赖
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.8.0</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.8.0</version>
</dependency>
2.配置
package com.yuyi.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springfox.documentation.builders.ApiInfoBuilder;
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
/**
* @EnableSwagger2 开启swagger2功能
*/
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket createRestApi() {
return new Docket(DocumentationType.SWAGGER_2).apiInfo(apiInfo()).select()
// api扫包范围
.apis(RequestHandlerSelectors.basePackage("com.yuyi.api")).paths(PathSelectors.any()).build();
}
//创建api文档信息
/**
* title 文档标题
* description 文档描述
*/
private ApiInfo apiInfo() {
return new ApiInfoBuilder().title("AkiraNicky").description("欢迎来到我的博客")
.termsOfServiceUrl("https://blog.csdn.net/AkiraNicky")
// .contact(contact)
.version("1.0").build();
}
}
3.实现
@Api("SwaggerDemo控制器")
@RestController
public class SwaggerController {
@ApiOperation("Swagger演示接口")
@GetMapping("/swaggerIndex")
public String swaggerIndex(){
System.out.println("swaggerIndex");
return "swaggerIndex";
}
@ApiOperation("单参数")
@ApiImplicitParam(name="userName",value="用户信息参数",required=true,dataType="String")
@PostMapping("/getMember")
public ResultBO<?> getMember(String userName){
return ResultTool.success(userName);
}
@ApiOperation("测试多参数")
@ApiResponses({ @ApiResponse(code = 200, message = "操作成功"),
@ApiResponse(code = 101, message = "查询内容为空"),
@ApiResponse(code = 102, message = "付款失败(微信支付)") })
@ApiImplicitParams({
@ApiImplicitParam(name="userName",value="用户名",required=true,dataType="String"),
@ApiImplicitParam(name="age",value="年龄",required=true,dataType="int")
})
@PostMapping("/testA")
public ResultBO<?> testA(String userName,Integer age){
Map<String,Object> map = new HashMap<>();
map.put("user_name", userName);
map.put("age", age);
if(age == 11){
return ResultTool.error(ZBExceptionEnum.EXCEPTION_NULL);
}
return ResultTool.success(map);
}
/***
* Swagger2自带调用方式,用不到postman
* http://localhost:9090/swagger-ui.html#/swagger-controller //9090为application.yml配的server.port
* <!-- 用springboot整合的swagger,不需要配置 -->
*/
}
4.启动项目,访问http://localhost:9090/swagger-ui.html
其中9090为项目的端口号,后面的地址为swagger的ui界面
5.Swagger使用的注解及其说明:
@Api:用在类上,说明该类的作用
@ApiOperation:注解来给API增加方法说明
@ApiImplicitParams : 一组参数说明
@ApiImplicitParam:单个参数说明
@ApiResponses:展示给前端的响应信息
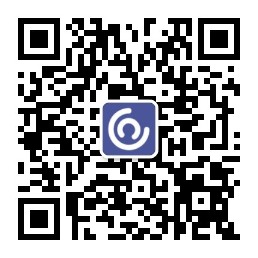
@ApiResponse:用在@ApiResponses中,一般用于表达一个错误的响应信息
code:数字,例如400
message:信息,例如"请求参数没填好"
response:抛出异常的类 ,一般用不到该参数
@ApiModel:描述一个Model的信息(一般用在请求参数无法使用@ApiImplicitParam注解进行描述的时候)
@ApiModelProperty:描述一个model的属性
方式二
这里介绍一个相当牛逼的工具,来自spring4all.com社区开源的swagger-spring-boot-starter。接下来我们就用这个依赖包开发swagger 文档。
1. 首先我 这里是个springcloud项目,在接口服务里引入以下依赖(springboot用的2.0.1版本,引入swagger1.8.0会报错):
<dependency>
<groupId>com.spring4all</groupId>
<artifactId>swagger-spring-boot-starter</artifactId>
<version>1.7.0.RELEASE</version>
</dependency>
2. 摒弃上面的SwaggerConfig配置,直接在application.yml进行配置:
swagger:
base-package: com.yuyi.controller
title: SpringCloud2.x构建微服务电商项目-会员服务接口
description: 基于SpringBoot+SpringCloud+Swagger+Docker+ELK+Kafka+Redis+RabbitMQ构建一套微服务电商项目
version: 1.0.1
terms-of-service-url: https://blog.csdn.net/AkiraNicky
contact:
name: bin
email: [email protected]
3. 启动类添加注解:@EnableSwagger2Doc
4.接下来就可以进行swagger自动生成api文档了,用法和上面的方式相同
@Api(tags = "微信服务接口")
public interface WxApi {
@ApiOperation(value = "获取wx基本信息")
@GetMapping("/getWx")
WxDO getWx();
}
方式三 (SpringCloud项目)
SpringCloud整合到Zuul网关可以进行做Swagger的统一管理
前提:每一个服务都得配方式二中的application.yml配置
1. 依赖(swagger 的依赖直接放在api接口中即可,不必放在实现里,并且@Api等注解统一写在api接口中)
<!-- 整合zuul -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-zuul</artifactId>
</dependency>
<!-- 整合swagger -->
<dependency>
<groupId>com.spring4all</groupId>
<artifactId>swagger-spring-boot-starter</artifactId>
<version>1.7.0.RELEASE</version>
</dependency>
2. application.yml配置
zuul:
routes:
api-a:
path: /api-wx/**
serviceId: shop-wx
api-order:
path: /api-member/**
serviceId: shop-member
3. ZuulApplication启动类
package com.yuyi;
import com.spring4all.swagger.EnableSwagger2Doc;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
import org.springframework.cloud.netflix.zuul.EnableZuulProxy;
import org.springframework.context.annotation.Primary;
import org.springframework.stereotype.Component;
import springfox.documentation.swagger.web.SwaggerResource;
import springfox.documentation.swagger.web.SwaggerResourcesProvider;
import java.util.ArrayList;
import java.util.List;
@SpringBootApplication
@EnableEurekaClient
@EnableSwagger2Doc
@EnableZuulProxy
public class ZuulApplication {
public static void main(String[] args) {
SpringApplication.run(ZuulApplication.class, args);
}
@Component
@Primary
class DocumentationConfig implements SwaggerResourcesProvider{
public List<SwaggerResource> get(){
List resources = new ArrayList<>();
// 网关使用服务别名获取远程服务的SwaggerAPI
// shop-wx可以随便写,/shop-wx/v2/api-docs必须以服务名开头
resources.add(swaggerResource("shop-wx", "/shop-wx/v2/api-docs", "2.0"));
resources.add(swaggerResource("shop-member", "/shop-member/v2/api-docs", "2.0"));
return resources;
}
private SwaggerResource swaggerResource(String name, String location, String version) {
SwaggerResource swaggerResource = new SwaggerResource();
swaggerResource.setName(name);
swaggerResource.setLocation(location);
swaggerResource.setSwaggerVersion(version);
return swaggerResource;
}
}
}
访问:http://localhost/swagger-ui.html,其中zuul端口号为80,即访问zuul的swagger:
、
注意:通过zuul进行swagger管理,访问的接口为zuul反向代理生成的地址: