一直困扰大家的问题,aidl的同步和异步调用的问题,在使用aidl的时候框架时, 所有服务的接口都是同步调用的,但是有些业务的需要异步调用服务的接口,到这里大家都束手无策了, 在这里提供一个修改方法的同步和异步调用的模板
客户端程序:
客户端主程序
package com.wytest.client;
import com.wytest.service.ICat;
import android.app.Activity;
import android.app.Service;
import android.content.ComponentName;
import android.content.Intent;
import android.content.ServiceConnection;
import android.os.Bundle;
import android.os.IBinder;
import android.os.RemoteException;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
/**
* @author [email protected]
* @version 1.0
*/
public class AidlClient extends Activity
{
private ICat catService;
private Button get;
EditText color, weight;
private ServiceConnection conn = new ServiceConnection()
{
@Override
public void onServiceConnected(ComponentName name, IBinder service)
{
// 获取远程Service的onBind方法返回的对象的代理
catService = ICat.Stub.asInterface(service);
}
@Override
public void onServiceDisconnected(ComponentName name)
{
catService = null;
}
};
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
get = (Button) findViewById(R.id.get);
color = (EditText) findViewById(R.id.color);
weight = (EditText) findViewById(R.id.weight);
// 创建所需绑定服务的Intent
Intent intent = new Intent();
intent.setAction("com.wytest.aidl.action.AIDL_SERVICE");
// 绑定远程服务
bindService(intent, conn, Service.BIND_AUTO_CREATE);
get.setOnClickListener(new OnClickListener()
{
@Override
public void onClick(View arg0)
{
try
{
// 获取、并显示远程Service的状态
color.setText(catService.getColor());
weight.setText(catService.getWeight() + "");
}
catch (RemoteException e)
{
e.printStackTrace();
}
}
});
}
@Override
public void onDestroy()
{
super.onDestroy();
// 解除绑定
this.unbindService(conn);
}
}
客户端封装的关于服务端的代理:
package com.wytest.service;
/**
* @author [email protected]
* @version 1.0
*/
public interface ICat extends android.os.IInterface {
/* Local-side IPC implementation stub class. /
public static abstract class Stub extends android.os.Binder implements
com.wytest.service.ICat {
private static final java.lang.String DESCRIPTOR = “org.crazyit.service.ICat”;
/** Construct the stub at attach it to the interface. */
public Stub() {
this.attachInterface(this, DESCRIPTOR);
}
/**
* Cast an IBinder object into an org.crazyit.service.ICat interface,
* generating a proxy if needed.
*/
public static com.wytest.service.ICat asInterface(
android.os.IBinder obj) {
if ((obj == null)) {
return null;
}
android.os.IInterface iin = obj.queryLocalInterface(DESCRIPTOR);
if (((iin != null) && (iin instanceof com.wytest.service.ICat))) {
return ((com.wytest.service.ICat) iin);
}
return new com.wytest.service.ICat.Stub.Proxy(obj);
}
@Override
public android.os.IBinder asBinder() {
return this;
}
@Override
public boolean onTransact(int code, android.os.Parcel data,
android.os.Parcel reply, int flags)
throws android.os.RemoteException {
switch (code) {
case INTERFACE_TRANSACTION: {
reply.writeString(DESCRIPTOR);
return true;
}
case TRANSACTION_getColor: {
data.enforceInterface(DESCRIPTOR);
java.lang.String _result = this.getColor();
reply.writeNoException();
reply.writeString(_result);
return true;
}
case TRANSACTION_getWeight: {
data.enforceInterface(DESCRIPTOR);
double _result = this.getWeight();
reply.writeNoException();
reply.writeDouble(_result);
return true;
}
}
return super.onTransact(code, data, reply, flags);
}
private static class Proxy implements com.wytest.service.ICat {
private android.os.IBinder mRemote;
Proxy(android.os.IBinder remote) {
mRemote = remote;
}
@Override
public android.os.IBinder asBinder() {
return mRemote;
}
public java.lang.String getInterfaceDescriptor() {
return DESCRIPTOR;
}
@Override
public java.lang.String getColor()
throws android.os.RemoteException {
android.os.Parcel _data = android.os.Parcel.obtain();
android.os.Parcel _reply = android.os.Parcel.obtain();
java.lang.String _result;
try {
_data.writeInterfaceToken(DESCRIPTOR);
mRemote.transact(Stub.TRANSACTION_getColor, _data, _reply, 1);
_reply.readException();
_result = _reply.readString();
} finally {
_reply.recycle();
_data.recycle();
}
return _result;
}
@Override
public double getWeight() throws android.os.RemoteException {
android.os.Parcel _data = android.os.Parcel.obtain();
android.os.Parcel _reply = android.os.Parcel.obtain();
double _result;
try {
_data.writeInterfaceToken(DESCRIPTOR);
mRemote.transact(Stub.TRANSACTION_getWeight, _data, _reply, 1);
_reply.readException();
_result = _reply.readDouble();
} finally {
_reply.recycle();
_data.recycle();
}
return _result;
}
}
static final int TRANSACTION_getColor = (android.os.IBinder.FIRST_CALL_TRANSACTION + 0);
static final int TRANSACTION_getWeight = (android.os.IBinder.FIRST_CALL_TRANSACTION + 1);
}
public java.lang.String getColor() throws android.os.RemoteException;
public double getWeight() throws android.os.RemoteException;
}
服务端程序:
服务端主程序:
/**
*
*/
package com.wytest.service;
import java.util.Timer;
import java.util.TimerTask;
import com.wytest.service.ICat.Stub;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import android.os.RemoteException;
import android.util.Log;
/**
* @author [email protected]
* @version 1.0
*/
public class AidlService extends Service
{
private CatBinder catBinder;
Timer timer = new Timer();
String[] colors = new String[]{
“红色”,
“黄色”,
“黑色”
};
double[] weights = new double[]{
2.3,
3.1,
1.58
};
private String color;
private double weight;
// 继承Stub,也就是实现额ICat接口,并实现了IBinder接口
public class CatBinder extends Stub
{
@Override
public String getColor() throws RemoteException
{
Log.v(“testaidl”, “getColor is called!!!”);
return color;
}
@Override
public double getWeight() throws RemoteException
{
Log.v(“testaidl”, “getWeight is callded!!!”);
return weight;
}
}
@Override
public void onCreate()
{
super.onCreate();
catBinder = new CatBinder();
timer.schedule(new TimerTask()
{
@Override
public void run()
{
// 随机地改变Service组件内color、weight属性的值。
int rand = (int)(Math.random() * 3);
color = colors[rand];
weight = weights[rand];
System.out.println(“——–” + rand);
}
} , 0 , 800);
}
@Override
public IBinder onBind(Intent arg0)
{
/* 返回catBinder对象
* 在绑定本地Service的情况下,该catBinder对象会直接
* 传给客户端的ServiceConnection对象
* 的onServiceConnected方法的第二个参数;
* 在绑定远程Service的情况下,只将catBinder对象的代理
* 传给客户端的ServiceConnection对象
* 的onServiceConnected方法的第二个参数;
*/
return catBinder;
}
@Override
public void onDestroy()
{
timer.cancel();
}
}
服务端的服务对象:
/*
* This file is auto-generated. DO NOT MODIFY.
* Original file: D:\android_project\AidlService\src\org\crazyit\service\ICat.aidl
*/
package com.wytest.service;
/**
* @author [email protected]
* @version 1.0
*/
public interface ICat extends android.os.IInterface {
/* Local-side IPC implementation stub class. /
public static abstract class Stub extends android.os.Binder implements
com.wytest.service.ICat {
private static final java.lang.String DESCRIPTOR = “org.crazyit.service.ICat”;
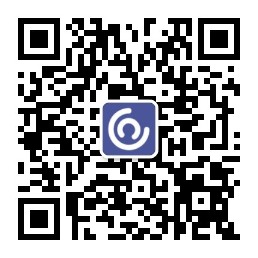
/** Construct the stub at attach it to the interface. */
public Stub() {
this.attachInterface(this, DESCRIPTOR);
}
/**
* Cast an IBinder object into an org.crazyit.service.ICat interface,
* generating a proxy if needed.
*/
@Override
public android.os.IBinder asBinder() {
return this;
}
@Override
public boolean onTransact(int code, android.os.Parcel data,
android.os.Parcel reply, int flags)
throws android.os.RemoteException {
switch (code) {
case INTERFACE_TRANSACTION: {
reply.writeString(DESCRIPTOR);
return true;
}
case TRANSACTION_getColor: {
data.enforceInterface(DESCRIPTOR);
java.lang.String _result = this.getColor();
reply.writeNoException();
reply.writeString(_result);
return true;
}
case TRANSACTION_getWeight: {
data.enforceInterface(DESCRIPTOR);
double _result = this.getWeight();
reply.writeNoException();
reply.writeDouble(_result);
return true;
}
}
return super.onTransact(code, data, reply, flags);
}
static final int TRANSACTION_getColor = (android.os.IBinder.FIRST_CALL_TRANSACTION + 0);
static final int TRANSACTION_getWeight = (android.os.IBinder.FIRST_CALL_TRANSACTION + 1);
}
public java.lang.String getColor() throws android.os.RemoteException;
public double getWeight() throws android.os.RemoteException;
}
注解.关于调用服务端方法决定同步和异步的代码是标红的部分,大家可以看一下试用aidl文件自动生成的代理对象与本文章生成的代理对象有何不同
源文件
aidl 修改后的aidl