目录
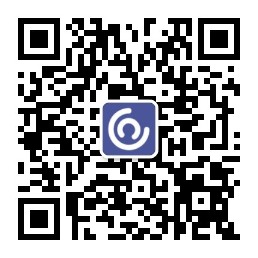
案例二:springsecurity+jwt +oauth2
案例3 springsecurity+jwt+oauth2+zull网关
动态权限控制
Rbac权限表设计
首先不管是实现哪种,都要基于rbac表设计,用户---中间表---角色---中间表---权限
案例1 springsecurity+ jwt
springsecurity是一个安全框架
jwt (JSON WEB Token )
优点:
- 无需再服务器存放用户的数据,减去服务器端压力
- 轻量级、json风格比较简单
- 跨语言
缺点:
Token一旦生成后期无法修改:
- 无法更新token有效期
- 无法销毁一个token
JWT组成:
第一部分:header (头部)
{
Typ=”jwt” ---类型为jwt
Alg:”HS256” --加密算法为hs256
}
第二部分:playload(载荷) 携带存放的数据 用户名称、用户头像之类 注意铭感数据
第三部分:secret (存放在服务器端)
Base64(header .playload) +秘钥
案例一实现步骤:
引入依赖
<dependencies>
<!-- SpringBoot整合Web组件 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
<!-- springboot整合freemarker -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
<!-->spring-boot 整合security -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<!-- springboot 整合mybatis框架 -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>1.3.2</version>
</dependency>
<!-- alibaba的druid数据库连接池 -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid-spring-boot-starter</artifactId>
<version>1.1.9</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.6.0</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.62</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
</dependency>
</dependencies>
创建UserEntity实体类
,用来实现UserDetails,注意有个属性权限数据
// 用户信息表
@Data
public class UserEntity implements UserDetails {
private Integer id;
private String username;
private String realname;
private String password;
private Date createDate;
private Date lastLoginTime;
private boolean enabled;
private boolean accountNonExpired;
private boolean accountNonLocked;
private boolean credentialsNonExpired;
// 用户所有权限
private List<GrantedAuthority> authorities = new ArrayList<GrantedAuthority>();
public Collection<? extends GrantedAuthority> getAuthorities() {
return authorities;
}
}
创建MemberUserDetailsService
实现UserDetailsService 接口,查询用户对应的权限放到authorities属性中,这样security,会自动获取到用户权限
@Component
@Slf4j
public class MemberUserDetailsService implements UserDetailsService {
@Autowired
private UserMapper userMapper;
/**
* loadUserByUserName
*
* @param username
* @return
* @throws UsernameNotFoundException
*/
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
// 1.根据该用户名称查询在数据库中是否存在
UserEntity userEntity = userMapper.findByUsername(username);
if (userEntity == null) {
return null;
}
// 2.查询对应的用户权限
List<PermissionEntity> listPermission = userMapper.findPermissionByUsername(username);
List<GrantedAuthority> authorities = new ArrayList<GrantedAuthority>();
listPermission.forEach(user -> {
authorities.add(new SimpleGrantedAuthority(user.getPermTag()));
});
log.info(">>>authorities:{}<<<", authorities);
// 3.将该权限添加到security
userEntity.setAuthorities(authorities);
return userEntity;
}
}
创建类SecurityConfig
,开启springsecurity,注解@EnableWebSecurity,然后进行一些列配置
@Component
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private MemberUserDetailsService memberUserDetailsService;
@Autowired
private PermissionMapper permissionMapper;
/**
* 添加授权账户
*
* @param auth
* @throws Exception
*/
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(memberUserDetailsService).passwordEncoder(new PasswordEncoder() {
/**
* 对密码MD5
* @param rawPassword
* @return
*/
@Override
public String encode(CharSequence rawPassword) {
return MD5Util.encode((String) rawPassword);
}
/**
* rawPassword 用户输入的密码
* encodedPassword 数据库DB的密码
* @param rawPassword
* @param encodedPassword
* @return
*/
public boolean matches(CharSequence rawPassword, String encodedPassword) {
String rawPass = MD5Util.encode((String) rawPassword);
boolean result = rawPass.equals(encodedPassword);
return result;
}
});
}
@Override
protected void configure(HttpSecurity http) throws Exception {
List<PermissionEntity> allPermission = permissionMapper.findAllPermission();
ExpressionUrlAuthorizationConfigurer<HttpSecurity>.ExpressionInterceptUrlRegistry
expressionInterceptUrlRegistry = http.authorizeRequests();
allPermission.forEach((permission) -> {
expressionInterceptUrlRegistry.antMatchers(permission.getUrl()).
hasAnyAuthority(permission.getPermTag());
});
expressionInterceptUrlRegistry.antMatchers("/auth/login").permitAll()
.antMatchers("/**").fullyAuthenticated()
// .and().formLogin().loginPage("/auth/login").and().csrf().disable();
.and()
// 设置过滤器
.addFilter(new JWTValidationFilter(authenticationManager()))
.addFilter(new JWTLoginFilter(authenticationManager())).csrf().disable()
// 剔除session
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS);
}
/**
* There is no PasswordEncoder mapped for the id "null"
* 原因:升级为Security5.0以上密码支持多中加密方式,恢复以前模式
*
* @return
*/
@Bean
public static NoOpPasswordEncoder passwordEncoder() {
return (NoOpPasswordEncoder) NoOpPasswordEncoder.getInstance();
}
}
创建JWTLoginFilter类
实现UsernamePasswordAuthenticationFilter接口,这个就是当springsecurity 验证成功以后,把token设置在header头中
public class JWTLoginFilter extends UsernamePasswordAuthenticationFilter {
/**
* 获取授权管理
*/
private AuthenticationManager authenticationManager;
public JWTLoginFilter(AuthenticationManager authenticationManager) {
this.authenticationManager = authenticationManager;
/**
* 后端登陆接口 为登陆接口
*/
super.setFilterProcessesUrl("/auth/login");
}
@Override
public Authentication attemptAuthentication(HttpServletRequest request,
HttpServletResponse response)
throws AuthenticationException {
// 调用我们MemberUserDetailsService 账号密码登陆
String username = this.obtainUsername(request);
String password = this.obtainPassword(request);
return authenticationManager.authenticate(
new UsernamePasswordAuthenticationToken(username,password,new ArrayList<>())
);
}
/**
* 账号和密码验证成功
*
* @param request
* @param response
* @param chain
* @param authResult
* @throws IOException
* @throws ServletException
*/
@Override
protected void successfulAuthentication(HttpServletRequest request, HttpServletResponse response,
FilterChain chain, Authentication authResult) throws IOException, ServletException {
// 账号和密码验证成功,在响应头中响应的jwt
UserEntity userEntity = (UserEntity) authResult.getPrincipal();
String jwtToken = MayiktJwtUtils.generateJsonWebToken(userEntity);
response.addHeader("token", jwtToken);
}
/**
* 账号密码验证失败
*
* @param request
* @param response
* @param failed
* @throws IOException
* @throws ServletException
*/
protected void unsuccessfulAuthentication(HttpServletRequest request, HttpServletResponse response, AuthenticationException failed) throws IOException, ServletException {
System.out.println("失败了");
}
}
用postman测试,登录成功token放入header中
案例二:springsecurity+jwt +oauth2
Oauth2.0 是一种开放的协议:核心:认证服务器,资源服务器
下面是一个流程图,
案例二实现步骤:
创建认证服务器
引入依赖
<dependencies>
<!-- https://mvnrepository.com/artifact/org.springframework.cloud/spring-cloud-starter-netflix-eureka-client -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.cloud/spring-cloud-starter-netflix-hystrix -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.cloud/spring-cloud-starter-netflix-ribbon -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-ribbon</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.cloud/spring-cloud-starter-openfeign -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/com.netflix.hystrix/hystrix-javanica -->
<dependency>
<groupId>com.netflix.hystrix</groupId>
<artifactId>hystrix-javanica</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.retry/spring-retry -->
<dependency>
<groupId>org.springframework.retry</groupId>
<artifactId>spring-retry</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-actuator -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-freemarker -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.data/spring-data-commons-core -->
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-commons</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.cloud/spring-cloud-security -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-security</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.cloud/spring-cloud-starter-oauth2 -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-oauth2</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.security/spring-security-jwt -->
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-jwt</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/mysql/mysql-connector-java -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-data-jpa -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.mybatis.spring.boot/mybatis-spring-boot-starter -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.projectlombok/lombok -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<scope>provided</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/com.alibaba/fastjson -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/com.alibaba/druid -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/io.springfox/springfox-swagger-ui -->
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/io.springfox/springfox-swagger2 -->
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
</dependency>
</dependencies>
创建实体类和实体类实现和上面的一样,直接复用
开启认证服务器,创建OauthServerConfig类,后面密码模式和授权码模式
@Configuration
@EnableAuthorizationServer
public class OauthServerConfig extends AuthorizationServerConfigurerAdapter {
//数据库连接池对象
@Autowired
private DataSource dataSource;
//认证业务对象
@Autowired
private AuthenticationManager authenticationManager;
//客户端信息对象
@Autowired
private ClientDetailsService clientDetailsService;
//存储策略
@Autowired
private TokenStore tokenStore;
//加密策略
@Autowired
private JwtAccessTokenConverter jwtAccessTokenConverter;
//从数据库中查询出客户端信息
@Bean
public JdbcClientDetailsService clientDetailsService() {
return new JdbcClientDetailsService(dataSource);
}
//token保存策略
@Bean
public TokenStore tokenStore() {
return new JdbcTokenStore(dataSource);
}
//授权信息保存策略
@Bean
public ApprovalStore approvalStore() {
return new JdbcApprovalStore(dataSource);
}
//授权码模式专用对象
@Bean
public AuthorizationCodeServices authorizationCodeServices() {
return new JdbcAuthorizationCodeServices(dataSource);
}
//指定客户端登录信息来源
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.withClientDetails(clientDetailsService());
}
//检查token的策略
// 授权服务器安全配置
@Override
public void configure(AuthorizationServerSecurityConfigurer oauthServer) throws Exception {
oauthServer.allowFormAuthenticationForClients();
oauthServer.checkTokenAccess("isAuthenticated()");
}
//OAuth2的配置信息
// 授权服务器端点配置
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints
// .approvalStore(approvalStore()) //开启密码模式
.authenticationManager(authenticationManager)
.authorizationCodeServices(authorizationCodeServices()) //开启授权码模式
.tokenServices(tokenServices()) //开启token令牌的 : jwt模式
.tokenStore(tokenStore()); // 开启token存储模式 : redis存储
}
/**
* 令牌管理模式
* @return
*/
@Bean
public AuthorizationServerTokenServices tokenServices(){
DefaultTokenServices defaultTokenServices = new DefaultTokenServices();
defaultTokenServices.setClientDetailsService(clientDetailsService);
defaultTokenServices.setSupportRefreshToken(true);
defaultTokenServices.setTokenStore(tokenStore);
// 令牌增强
TokenEnhancerChain tokenEnhancerChain = new TokenEnhancerChain();
tokenEnhancerChain.setTokenEnhancers(Arrays.asList(jwtAccessTokenConverter));
defaultTokenServices.setTokenEnhancer(tokenEnhancerChain);
defaultTokenServices.setAccessTokenValiditySeconds(7200); // 两小时
defaultTokenServices.setRefreshTokenValiditySeconds(259200); // 3天
return defaultTokenServices;
}
}
开启 springsecurity 安全框架,创建WebSecurityConfig2类
@Configuration
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled = true)
public class WebSecurityConfig2 extends WebSecurityConfigurerAdapter {
// @Autowired
// private UserService userService;
@Autowired
private UserDetailsServiceImpl userDetailsService;
//设置权限
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.loginProcessingUrl("/auth/login")
.permitAll()
.and()
.csrf()
.disable();
}
//注入PasswordEncoder
//采用bcrypt对密码进行编码
@Bean
public PasswordEncoder passwordEncoder(){
return new BCryptPasswordEncoder();
}
//指定认证对象的来源
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder());
}
//AuthenticationManager对象在Oauth2认证服务中要使用,提取放到IOC容器中
@Override
@Bean
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
}
开启令牌策略。创建TokenConfig类,我用的Hs256 加密,我注释的使用RSA实现非对称加密
@Configuration
public class TokenConfig {
@Autowired
private RedisConnectionFactory redisConnectionFactory;
/**
* 令牌存储策略
* @return
*/
@Bean
public TokenStore tokenStore(){
RedisTokenStore redisTokenStore = new RedisTokenStore(redisConnectionFactory);
redisTokenStore.setPrefix("token"+ GlobalsConstants.OAUTH_PREFIX);
return redisTokenStore;
// return new JwtTokenStore(accessTokenConverter());
}
/**
* 加密策略 Hs256
* @return
*/
@Bean
public JwtAccessTokenConverter accessTokenConverter(){
JwtAccessTokenConverter jwtAccessTokenConverter = new JwtAccessTokenConverter();
// 密钥加密 “dyc"
jwtAccessTokenConverter.setSigningKey("dyc");
return jwtAccessTokenConverter;
}
///==========================================================分割线===============================
// /**
// * 读取密钥配置
// * @return
// */
// @Bean("keyProp")
// public KeyProperties keyProperties(){
// return new KeyProperties();
// }
//
// @Resource(name = "keyProp")
// private KeyProperties keyProperties;
/**
* 使用RSA非对称加密算法来对Token进行签名
* @return
*/
// @Bean
// public JwtAccessTokenConverter jwtAccessTokenConverter(CustomUserAuthenticationConverter customUserAuthenticationConverter) {
// JwtAccessTokenConverter converter = new JwtAccessTokenConverter();
// // 导入证书
// KeyPair keyPair = new KeyStoreKeyFactory
// (keyProperties.getKeyStore().getLocation(), keyProperties.getKeyStore().getSecret().toCharArray())
// .getKeyPair(keyProperties.getKeyStore().getAlias(),keyProperties.getKeyStore().getPassword().toCharArray());
// converter.setKeyPair(keyPair);
// //配置自定义的CustomUserAuthenticationConverter
DefaultAccessTokenConverter accessTokenConverter = (DefaultAccessTokenConverter) converter.getAccessTokenConverter();
accessTokenConverter.setUserTokenConverter(customUserAuthenticationConverter);
// return converter;
// }
///==========================================================分割线===============================
// @Bean
// public JwtAccessTokenConverter accessTokenConverter() {
// JwtAccessTokenConverter jwtAccessTokenConverter = new JwtAccessTokenConverter();
// jwtAccessTokenConverter.setKeyPair(keyPair());
// return jwtAccessTokenConverter;
// }
//
// /**
// * 生成密钥 : keytool -genkey -alias jwt -keyalg RSA -keystore jwt.jks
// * @return
// */
// @Bean
// public KeyPair keyPair() {
// //从classpath下的证书中获取秘钥对
// KeyStoreKeyFactory keyStoreKeyFactory = new KeyStoreKeyFactory(new ClassPathResource("jwt.jks"), "123456".toCharArray());
// // 123456是jwt的密钥
// return keyStoreKeyFactory.getKeyPair("jwt", "123456".toCharArray());
// }
}
创建资源服务器项目,
其实资源服务器中的配置很简单,就是在认证服务器怎么加密的token,在认证服务器用同样的密钥 给解token,获取用户的权限,再根据权限获取用户数据
开启资源服务器,创建类ResourceServerConfig
@Configuration
@EnableResourceServer
public class ResourceServerConfig extends ResourceServerConfigurerAdapter {
public static final String RESOURCE_ID = "order";
@Autowired
TokenStore tokenStore;
@Override
public void configure(ResourceServerSecurityConfigurer resources) throws Exception {
resources.resourceId((RESOURCE_ID))
.tokenStore(tokenStore)
.stateless(true);
}
@Override
public void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/**").access("#oauth2.hasScope('all')")
.and()
.csrf().disable()
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS);
}
*/
/*
@Bean
public ResourceServerTokenServices tokenServices(){
RemoteTokenServices remoteTokenServices = new RemoteTokenServices();
remoteTokenServices.setCheckTokenEndpointUrl("http://localhost:8080/uaa/auth/check_token");
remoteTokenServices.setClientId("c1");
remoteTokenServices.setClientSecret("secret");
return remoteTokenServices;
}
*//*
}
创建TokenConfig类,设置token解密,注意密钥 一定要和 认证服务器的一样才能解token
@Configuration
public class TokenConfig {
private final String KEY_PAIR = "dyc";
/**
* 令牌存储策略
* @return
*/
@Bean
public TokenStore tokenStore(){
return new JwtTokenStore(accessTokenConverter());
}
@Bean
public JwtAccessTokenConverter accessTokenConverter(){
JwtAccessTokenConverter jwtAccessTokenConverter = new JwtAccessTokenConverter();
//jwtAccessTokenConverter.setKeyPair(KEY_PAIR);
jwtAccessTokenConverter.setSigningKey(KEY_PAIR);
return jwtAccessTokenConverter;
}
}
密码模式测试:
访问 : localhost:11000/auth/oauth/authorize?client_id=jrkj&response_type=code
点击授权后,获取,授权码
获取token,用postman测试,返回token
授权码模式测试,
直接带着账户密码,请求之后获取到了token:
案例3 springsecurity+jwt+oauth2+zull网关
整合网关的话,其实认证服务上的代码是没有改变的,改变的时候资源服务器和网关中的代码,
资源服务器项目中的验证token的功能要放到网关去,在网关统一做token验证,然后解析token!后,根据权限来请求微服务中的数据,
这块就贴个图了:
网关中的代码:
创建ResourceServerConfig类,开启资源服务器
@Configuration
@EnableResourceServer
public class ResourceServerConfig {
public static final String RESOURCE_ID = "zuul";
//----------------------------------------------------------分割线--------------------------------
// order资源
@Configuration
@EnableResourceServer
public class OrderServerConfig extends ResourceServerConfigurerAdapter{
@Autowired
TokenStore tokenStore;
@Override
public void configure(ResourceServerSecurityConfigurer resources) throws Exception {
resources.tokenStore(tokenStore).resourceId(RESOURCE_ID)
.stateless(true);
}
@Override
public void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
// .antMatchers("/resource/**").access("#oauth2.hasScope('ROLE_API')");
.antMatchers("/resource/**").permitAll();
}
}
//----------------------------------------------------------分割线--------------------------------
// uaa资源
@Configuration
@EnableResourceServer
public class UAAServerConfig extends ResourceServerConfigurerAdapter{
@Autowired
TokenStore tokenStore;
@Override
public void configure(ResourceServerSecurityConfigurer resources) throws Exception {
resources.tokenStore(tokenStore).resourceId(RESOURCE_ID)
.stateless(true);
}
@Override
public void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/auth/**").permitAll();
}
}
//----------------------------------------------------------分割线--------------------------------
}
创建SecurityConfig类,开启springsecurity安全验证
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/**").permitAll()
.and().csrf().disable();
}
}
创建TokenConfig类,解密token
@Configuration
public class TokenConfig {
// token 加密的密钥
private final String KEY_PAIR = "dyc";
/**
* 令牌存储策略
* @return
*/
@Bean
public TokenStore tokenStore(){
return new JwtTokenStore(accessTokenConverter());
}
@Bean
public JwtAccessTokenConverter accessTokenConverter(){
JwtAccessTokenConverter jwtAccessTokenConverter = new JwtAccessTokenConverter();
//jwtAccessTokenConverter.setKeyPair(KEY_PAIR);
jwtAccessTokenConverter.setSigningKey(KEY_PAIR);
return jwtAccessTokenConverter;
}
}