Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Total Submission(s): 350199 Accepted Submission(s): 83372
Problem Description
Given a sequence a[1],a[2],a[3]…a[n], your job is to calculate the max sum of a sub-sequence. For example, given (6,-1,5,4,-7), the max sum in this sequence is 6 + (-1) + 5 + 4 = 14.
Input
The first line of the input contains an integer T(1<=T<=20) which means the number of test cases. Then T lines follow, each line starts with a number N(1<=N<=100000), then N integers followed(all the integers are between -1000 and 1000).
Output
For each test case, you should output two lines. The first line is “Case #:”, # means the number of the test case. The second line contains three integers, the Max Sum in the sequence, the start position of the sub-sequence, the end position of the sub-sequence. If there are more than one result, output the first one. Output a blank line between two cases.
Sample Input
2
5 6 -1 5 4 -7
7 0 6 -1 1 -6 7 -5
Sample Output
Case 1:
14 1 4
Case 2:
7 1 6
问题链接:HDU1003 Max Sum
问题简述:(略)
问题分析:
动态规划的的最大子段和问题,参见参考链接。这个问题跟参考链接基本上相同,只是输入输出不同,需要输出最大子段和的起始和终止序号。
还有一种解决方法是滑动窗口法。
程序说明:(略)
参考链接:HDU1231 最大连续子序列
题记:(略)
AC的C++语言程序(滑动窗口法)如下:
/* HDU1003 Max Sum */
#include <bits/stdc++.h>
using namespace std;
const int N= 100000 + 1;
int a[N];
int main()
{
int t, n;
scanf("%d", &t);
for(int k = 1; k <= t; k++) {
scanf("%d", &n);
for(int i = 1; i <= n; i++) scanf("%d", &a[i]);
// 滑动窗口法
int maxSum = INT_MIN, subSum = 0, mstart, mend, l = 1;
for(int i = 1; i <= n; i++) {
subSum += a[i];
if(subSum > maxSum)
maxSum = subSum, mstart = l, mend = i;
if(subSum < 0)
subSum = 0, l = i + 1;
}
printf("Case %d:\n", k);
printf("%d %d %d\n", maxSum, mstart, mend);
if(k != t) printf("\n");
}
return 0;
}
AC的C++语言程序如下:
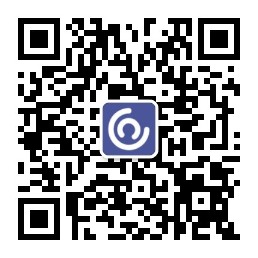
/* HDU1003 Max Sum */
#include <bits/stdc++.h>
using namespace std;
const int N= 100000;
int a[N], maxSum, mstart, mend;
void maxSubSum(int n)
{
int subSum, sstart;
maxSum = a[0], mstart = mend = sstart = 1;
subSum = 0;
for(int i = 0; i<n; i++) {
if(subSum < 0) subSum = a[i], sstart = i + 1;
else subSum += a[i];
if(subSum > maxSum)
maxSum = subSum, mstart = sstart, mend = i + 1;
}
}
int main()
{
int t, n;
scanf("%d", &t);
for(int k = 1; k <= t; k++) {
scanf("%d", &n);
for(int i = 0; i < n; i++) scanf("%d", &a[i]);
maxSubSum(n);
printf("Case %d:\n", k);
printf("%d %d %d\n", maxSum, mstart, mend);
if(k != t) printf("\n");
}
return 0;
}
AC的C++语言程序如下:
/* HDU1003 Max Sum */
#include <bits/stdc++.h>
using namespace std;
int main()
{
// max, mstart, mend为一组,是已经求得的最大子段和
// sum, sstart, j为一组,是当前正在进行计算的最大子段和
// 当前的子段不再单调增大时,则重新开启一个新的子段
int t, n, maxSum, mstart, mend, subSum, sstart, cur;
scanf("%d", &t);
for(int k = 1; k <= t; k++) {
scanf("%d", &n);
// 一个元素时,它就是目前的最大子段和;最大子段和的起始和终止分别是maxstart和end
scanf("%d", &cur);
maxSum = subSum = cur;
mstart = mend = sstart = 1;
for(int j = 2; j <= n; j++) {
scanf("%d", &cur);
if(subSum < 0)
// 不单调递增时(之前子段和为负),把当前的元素预存为另外的一个子段
subSum = cur, sstart = j;
else
// 单调递增
subSum += cur;
// 当前正在进行计算的最大子段和超过之前的最大子段和,则重置最大子段和
if(subSum > maxSum)
maxSum = subSum, mstart = sstart, mend = j;
}
printf("Case %d:\n", k);
printf("%d %d %d\n", maxSum, mstart, mend);
if(k != t) printf("\n");
}
return 0;
}