Musical Theme
Time Limit: 1000MS | Memory Limit: 30000K | |
Total Submissions: 33820 | Accepted: 11259 |
Description
A musical melody is represented as a sequence of N (1<=N<=20000)notes that are integers in the range 1..88, each representing a key on the piano. It is unfortunate but true that this representation of melodies ignores the notion of musical timing; but, this programming task is about notes and not timings.
Many composers structure their music around a repeating &qout;theme&qout;, which, being a subsequence of an entire melody, is a sequence of integers in our representation. A subsequence of a melody is a theme if it:
Transposed means that a constant positive or negative value is added to every note value in the theme subsequence.
Given a melody, compute the length (number of notes) of the longest theme.
One second time limit for this problem's solutions!
Many composers structure their music around a repeating &qout;theme&qout;, which, being a subsequence of an entire melody, is a sequence of integers in our representation. A subsequence of a melody is a theme if it:
- is at least five notes long
- appears (potentially transposed -- see below) again somewhere else in the piece of music
- is disjoint from (i.e., non-overlapping with) at least one of its other appearance(s)
Transposed means that a constant positive or negative value is added to every note value in the theme subsequence.
Given a melody, compute the length (number of notes) of the longest theme.
One second time limit for this problem's solutions!
Input
The input contains several test cases. The first line of each test case contains the integer N. The following n integers represent the sequence of notes.
The last test case is followed by one zero.
The last test case is followed by one zero.
Output
For each test case, the output file should contain a single line with a single integer that represents the length of the longest theme. If there are no themes, output 0.
Sample Input
30 25 27 30 34 39 45 52 60 69 79 69 60 52 45 39 34 30 26 22 18 82 78 74 70 66 67 64 60 65 80 0
Sample Output
5
思路:
py大佬太强了!!!!
这个题可以算是hash的模板题了,不知道学成这个样子有没有算是入门,接下来就是直接上代码了。。。
emmmm,还有很多题解说是后缀数组,可是我并不知道那是什么东西,等一下去学一下好了。
这份代码是照着py大佬的代码打的:
http://www.cnblogs.com/qldabiaoge/p/9152430.html
代码(Hash 散列表)
#include<iostream> #include<cstdio> #include<cstring> using namespace std; typedef unsigned long long ll; const int maxn = 20010,Hash = 10007; /**如果我的推理没有错的话。。。。 这题可以不搞这个结构体呀 为什么不用map,或者是直接用结构体数组二分查找?? **/ struct hashmap//这个玩意只维护了特定长度的序列的值 { int head[maxn],next[maxn],siz,f[maxn]; ll state[maxn]; void init()//初始化 { siz=0;//siz就是散列表的大小呀 memset(head,-1,sizeof(head)); } int add(unsigned long long val,int _id)//将某个值与他的标号加入散列表? {//好像是一个模拟链表诶 int h=val % Hash;//h模掉hash,作为一个粗略的hash值,加速查询 //h貌似只是用来查询一个引子 for(int i=head[h];i!=-1;i=next[i]){// if(val==state[i]){return f[i];}//state存的应该就是值了,那么f[i]存的就是出现这个值的 //第一个位置了。 } f[siz]=_id;//存下标 state[siz]=val;//存值 next[siz]=head[h];//维护查询路线 head[h]=siz++; return f[siz-1];//返回首次出现的此hash值的位置 } }H; const int seed = 13331; ll p[maxn],s[maxn]; int a[maxn],n; int check(int x)//x是子串的长度 { H.init();//每检查一次,就初始化一次 //可能情况太多,内存达不到要求,所以只能一段长度更新一次。 //而将其注释后超时其实是因为数组越界造成的无限循环 for(int i=x;i<n;i++){ if(H.add(s[i]-s[i-x]*p[x],i)<i-x)return 1;//s[i]的值,由a[1]到a[i]决定 //s[i-x]的值,由a[1]到a[i-x]决定; //就是找出当前的值是否在之前出现过,并且没有重叠 //如何证明s[i]-s[i-x]*p[x]对于每一完全一致的字串,他们的值相等 }return 0; } int main() { p[0]=1; for(int i=1;i<maxn;i++){ p[i]=p[i-1]*seed; }//进制数组 while(scanf("%d",&n)&&n){ for(int i=1;i<=n;i++){ scanf("%d",&a[i]); }//输入 for(int i=1;i<n;i++){ a[i]=a[i+1]-a[i]; }//处理两个数之间的差值 for(int i=1;i<n;i++){ s[i]=s[i-1]*seed+a[i];//a[i]是原始的差值,s[i]应该是一个与a[i]有关,而没有具体意义的数组· //将s[i]展开,就是∑a[i]*seed^(i-1) } int ans=0; int low=4,high=n-1;//二分答案 while(low<=high){ int mid = (low+high)>>1; if(check(mid)){//check检查答案是否可行 ans=mid; low=mid+1; } else high = mid-1; } if(ans<4){ans=-1;} printf("%d\n",ans+1); } return 0; }
Thanks♪(・ω・)ノ
接下来是第48处的证明
我把思路写一下。
s[i]数组与a[i]的值与i的值有着密切的关系,根据代码你可以将某一个s[n]展开
同时p[x]你也展开
假设x>y: s[x]-s[y]*p[x-y]
扫描二维码关注公众号,回复:
2649091 查看本文章
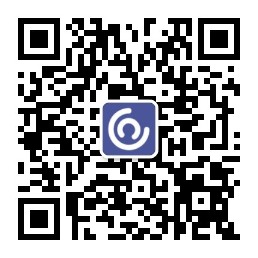
全部展开一下,整理,得到的式子,你会发现,它的值只与每一个a[i]的值有关,而它的位置对值产生的影响,已经在这个式子中被减去为0了。
所以,如果是两个完全一样的字串,无论在那个位置,通过这个式子算出来的值都会相等。
再说一下,这个散列表确实比较骚,不过每次查找表中之前有没有出现相同的hash值时,最坏的情况可能是遍历了一遍,因为hash值是0--2^64,就是相当于10的18次方,那么获得相同h的hash值共有10的18次方/Hash。也就是10的14次方个。。。不过这种情况应该是不可能出现的,或许在程序设计竞赛中,根本不会出现这种情况。