Goal
In this tutorial you will learn:
- What an image histogram is and why it is useful
- 什么是图像的直方图,它有什么用?
- To equalize histograms of images by using the OpenCV function cv::equalizeHist
- 使用OpenCV函数cv::equalizeHist进行图像直方图的均衡化
Theory
What is an Image Histogram?
- It is a graphical representation of the intensity distribution of an image.
- 图像直方图代表了图像中像素的分布
- It quantifies the number of pixels for each intensity value considered.
- 它量化了每个像素值的数量
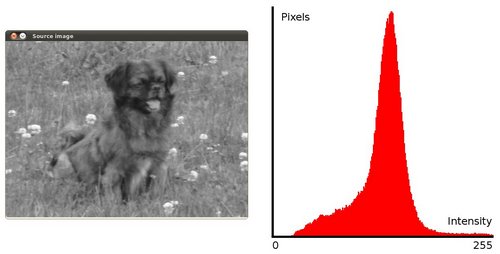
What is Histogram Equalization?
- It is a method that improves the contrast in an image, in order to stretch out the intensity range.
- 它是一种方法提高图像的对比图,为了扩展像素值的范围。
- To make it clearer, from the image above, you can see that the pixels seem clustered around the middle of the available range of intensities. What Histogram Equalization does is to stretch out this range. Take a look at the figure below: The green circles indicate the underpopulated intensities. After applying the equalization, we get an histogram like the figure in the center. The resulting image is shown in the picture at right.
- 为了更加清晰得说明这一点,你可以看到在下图中,像素值主要聚集在中间的几个刻度上。直方图均衡化就是扩展这个范围。如下图所示。绿色圆表明了这些是不受欢迎的像素值。使用均衡化后,可以得到的直方图如中间这个图所示。图片的结果如右图所示。
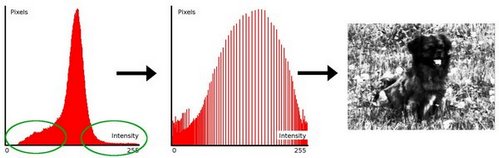
How does it work?
- Equalization implies mapping one distribution (the given histogram) to another distribution (a wider and more uniform distribution of intensity values) so the intensity values are spread over the whole range.
- 直方图均衡化意味这重新映射了像素分布到另外一个像素分布,以使得像素值分布在整个范围内。
To accomplish the equalization effect, the remapping should be the cumulative distribution function (cdf) (more details, refer to Learning OpenCV). For the histogram H(i), its cumulative distribution H′(i) is:
为了完成这一点,重映射是累计映射函数。比如直方图H(i),那么它的累积函数应该为H(i)如下:
H′(i)=∑0≤j<iH(j)To use this as a remapping function, we have to normalize H′(i) such that the maximum value is 255 ( or the maximum value for the intensity of the image ). From the example above, the cumulative function is:
使用这个作为映射函数,我们需要对其进行归一化,这样最大值为255(或者像素的最大值)。这样累计函数就是
Finally, we use a simple remapping procedure to obtain the intensity values of the equalized image:
最后我们使用一个简单的重映射程序获得均衡化图像的像素值。
equalized(x,y)=H′(src(x,y))
Code
- What does this program do?
- Loads an image(加载图像)
- Convert the original image to grayscale(转变为灰度图)
- Equalize the Histogram by using the OpenCV function cv::equalizeHist(使用这个函数)
- Display the source and equalized images in a window.(显示原图像和后来的图像)
#include "opencv2/imgcodecs.hpp"
#include "opencv2/highgui.hpp"
#include "opencv2/imgproc.hpp"
#include <iostream>
using namespace cv;
using namespace std;
int main( int argc, char** argv )
{
Mat src, dst;
const char* source_window = "Source image";
const char* equalized_window = "Equalized Image";
CommandLineParser parser( argc, argv, "{@input | lena.jpg | input image}" );
src = imread( parser.get<String>( "@input" ), IMREAD_COLOR );
if( src.empty() )
{
cout << "Could not open or find the image!\n" << endl;
cout << "Usage: " << argv[0] << " <Input image>" << endl;
return -1;
}
cvtColor( src, src, COLOR_BGR2GRAY );
equalizeHist( src, dst );
namedWindow( source_window, WINDOW_AUTOSIZE );
namedWindow( equalized_window, WINDOW_AUTOSIZE );
imshow( source_window, src );
imshow( equalized_window, dst );
waitKey(0);
return 0;
}
代码是如此的简单,就不再解释了:
CMakeLists.txt
cmake_minimum_required(VERSION 2.8)
project( DisplayImage )
find_package( OpenCV REQUIRED )
include_directories( ${OpenCV_INCLUDE_DIRS} )
add_executable( DisplayImage main.cpp )
target_link_libraries( DisplayImage ${OpenCV_LIBS} )
install(TARGETS DisplayImage RUNTIME DESTINATION bin