目录
前端使用Vue.js+Element.UI开发,后端使用Springboot+MyBatis。数据库方面使用MySQL。需要源码可加Q:914259961
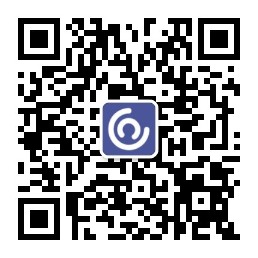
2.1、前端模块
登录页面由一个表单构成,有两个输入框,账号和密码,输入成功后跳转到信息管理页面,否则提示登录失败的原因。
然后信息管理页面有四个,分别是学生信息管理,班级信息管理,教师信息管理,课程信息管理。
学生信息管理页面可以对学生的信息进行管理,并可以通过学生的姓名查询,以及通过班级区分。
班级信息管理页面可以对班级的信息进行管理,可以通过专业进行查询。双击班级可以跳转到该班级的所有学生页面。
教师信息管理页面可以对教师的信息进行管理,可以通过教师的名称查询。
课程信息管理页面可以对课程信息进行管理,可以通过课程名进行查询,并通过课程开设学期,班级,授课教师进行区分。
双击学期单元格会跳转到该学期的所有课程;双击班级会跳转到该班级的所有课程;双击教师会跳转到以该教师的所有课程。可以同时选择。
2.2、后端模块
表现层用来接受数据和返回数据。采用RestFul接口风格,通过接口的类别区分接口的功能。
服务层是具体业务实现的地方。对表现层的数据进行处理,并调用持久层的接口,对数据进行增删查改以及进行相关的级联操作。
因为数据库方面采用的是逻辑外键,需要在服务层对表与表之间的关联进行相关操作。
持久层是访问数据的地方。采用了MyBatis框架,对数据库的访问进行了封装,通过关联数据库表相关的实体,完成对象关系映射,简化了数据库的操作。
图1 类图
2.3、数据库模块
数据库采用MySQL,库名为student_db。一共建有六个表,分别为学生表,教师表,班级表,课程表,账号表以及flyway自动构建数据库的历史记录表。
在学生表中添加了班级表的外键,然后在课程表中添加了班级表的外键和教师表的外键。通过这些外键关联,完成的后端模块中的实体之间的关联,
使得其在后端中的使用更加便捷。
图2 数据库表结构
3.1、前端实现
1、登录页
页面正中间为一个表单,两个输入框添加了表单验证,不能为空,然后两个按钮一个是登录,一个是重置掉输入框的输入。表单采用了透明化处理。
图3 登录页
2、学生管理页
图4 学生管理页
图5 添加学生
图6 编辑学生
3、班级管理页
图7 班级管理页
图8 添加班级
图9 编辑班级
4、教师管理页
图10 教师管理页
图11 添加教师
图12 编辑教师
5、课程管理页
图13 课程管理页
图14 添加课程
图15 编辑课程
3.2、后端实现
1、程序入口
package com.management.app;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@MapperScan("com.management.app.core.mapper")
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
2、表现层(学生信息)
package com.management.app.api;
import com.management.app.core.model.entity.Student;
import com.management.app.core.service.IStudentService;
import com.management.app.support.Rest;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/students")
public class StudentApi {
private final IStudentService service;
public StudentApi(IStudentService service) {
this.service = service;
}
@GetMapping
public Rest<?> findPaging(@RequestParam(required = false) Integer pageNumber,
@RequestParam(required = false) Integer pageSize,
@RequestParam(required = false) String name,
@RequestParam(required = false) Integer teamId) {
return Rest.ok(service.findPaging(pageNumber, pageSize, teamId, name));
}
@GetMapping("/{id}")
public Rest<Student> findById(@PathVariable Integer id) {
return Rest.ok(service.findById(id));
}
@PostMapping
public Rest<?> create(@RequestBody Student student) {
service.create(student);
return Rest.ok();
}
@PutMapping("/{id}")
public Rest<?> update(@PathVariable Integer id, @RequestBody Student student) {
service.update(id, student);
return Rest.ok();
}
@DeleteMapping("/{id}")
public Rest<?> delete(@PathVariable Integer id) {
service.delete(id);
return Rest.ok();
}
}
3、服务层(学生信息)
package com.management.app.core.service.impl;
import com.management.app.core.model.entity.Student;
import com.management.app.core.mapper.StudentMapper;
import com.management.app.core.model.vo.StudentVO;
import com.management.app.core.service.IStudentService;
import com.management.app.support.PageInfo;
import org.springframework.stereotype.Service;
import java.util.List;
import static com.management.app.infrastructure.consts.AppConst.DEFAULT_NUMBER;
import static com.management.app.infrastructure.consts.AppConst.DEFAULT_SIZE;
@Service
public class StudentServiceImpl implements IStudentService {
private final StudentMapper mapper;
public StudentServiceImpl(StudentMapper mapper) {
this.mapper = mapper;
}
@Override
public Student findById(Integer id) {
return mapper.selectStudent(id);
}
@Override
public PageInfo findPaging(Integer pageNumber, Integer pageSize,Integer teamId, String name) {
Integer totalCount = mapper.selectStudentCount(teamId, name);
if (pageNumber == null || pageSize == null) {
// 默认返回分页8
return new PageInfo(DEFAULT_NUMBER,
DEFAULT_SIZE,
totalCount,
mapper.selectStudentPage(DEFAULT_NUMBER, DEFAULT_SIZE, teamId, name));
}
List<Student> content = mapper.selectStudentPage(pageNumber * pageSize, pageSize, teamId, name);
List<StudentVO> vos = StudentVO.mapFrom(content);
return new PageInfo(pageNumber, pageSize, totalCount, vos);
}
private void setBaseProperties(Student entity, Student model) {
entity.setProperties(model);
}
@Override
public void create(Student model) {
Student entity = new Student();
setBaseProperties(entity, model);
mapper.insertStudent(entity);
}
@Override
public void update(Integer id, Student model) {
Student entity = findById(id);
setBaseProperties(entity, model);
mapper.updateStudent(entity);
}
@Override
public void delete(Integer id) {
mapper.deleteStudent(id);
}
}
4、持久层(学生信息)
package com.management.app.core.mapper;
import com.management.app.core.model.entity.Student;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public interface StudentMapper {
Student selectStudent(Integer id);
Integer selectStudentCount(Integer teamId, String name);
List<Student> selectStudentPage(Integer startIndex, Integer pageSize, Integer teamId, String name);
void insertStudent(Student student);
void updateStudent(Student student);
void deleteStudent(Integer id);
void deleteStudents(Integer teamId);
}
Mapper.xml:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.management.app.core.mapper.StudentMapper">
<resultMap id="studentMap" type="Student">
<result column="id" property="id"/>
<result column="name" property="name"/>
<result column="student_number" property="studentNumber"/>
<result column="birth_date" property="birthDate" />
<result column="gender" property="gender" />
<result column="national" property="national" />
<result column="phone_number" property="phoneNumber" />
<result column="native_place" property="nativePlace"/>
<result column="team_id" property="teamId"/>
<!-- <association property="team" column="team_id"-->
<!-- resultMap="teamMap" columnPrefix="t_"/>-->
</resultMap>
<!--使用嵌套查询,可避免n+1问题-->
<select id="selectStudent" resultMap="studentMap">
select
id,
name,
student_number,
birth_date,
gender,
national,
phone_number,
team_id,
native_place
from student
where id = #{id};
</select>
<!-- 查询总个数 (只有一个参数时用_parameter) 使用CONCAT拼接%通配符 -->
<select id="selectStudentCount" resultType="java.lang.Integer">
select count(*)
from student
<if test="teamId!=null">where team_id = #{teamId}
<if test="name!=null">and name like CONCAT('%',#{name},'%')</if>
</if>
<if test="teamId==null">
<if test="name!=null">where name like CONCAT('%',#{name},'%')</if>
</if>
</select>
<select id="selectStudentPage" resultMap="studentMap">
select *
from student
<if test="teamId!=null">where team_id = #{teamId}
<if test="name!=null">and name like CONCAT('%',#{name},'%')</if>
</if>
<if test="teamId==null">
<if test="name!=null">where name like CONCAT('%',#{name},'%')</if>
</if>
order by CONVERT(student_number,SIGNED) asc
limit #{startIndex},#{pageSize}
</select>
<insert id="insertStudent" parameterType="com.management.app.core.model.entity.Student" keyProperty="id">
insert into
student(name, student_number, birth_date, gender, national, phone_number, team_id, native_place)
values(#{name}, #{studentNumber}, #{birthDate}, #{gender}, #{national}, #{phoneNumber}, #{teamId}, #{nativePlace});
</insert>
<update id="updateStudent" parameterType="com.management.app.core.model.entity.Student">
UPDATE student
<set>
<if test="name != null">name=#{name},</if>
<if test="studentNumber!=null">student_number=#{studentNumber},</if>
<if test="birthDate!=null">birth_date=#{birthDate},</if>
<if test="gender!=null">gender=#{gender},</if>
<if test="national!=null">national=#{national},</if>
<if test="phoneNumber!=null">phone_number=#{phoneNumber},</if>
<if test="teamId!=null">team_id=#{teamId},</if>
<if test="nativePlace!=null">native_place=#{nativePlace},</if>
</set>
WHERE
id=#{id};
</update>
<delete id="deleteStudent" parameterType="int" >
delete from
student
where
id=#{id}
</delete>
<delete id="deleteStudents" parameterType="int" >
delete from
student
where
team_id=#{teamId}
</delete>
</mapper>