unity对象生命周期函数
Awake():最早调用,所以可以实现单例模式
OnEnable():组件激活后调用,在Awake后调用一次
Stat():在Update()之前,OnEnable()之后调用,可以在此设置一些初始值
Update():帧率调用方法,每帧调用一次,每次调用与上次调用时间间隔不相同
FixeUpdate():固定频率调用方法,每次调用与上次调用时间间隔相同
LateUpdate():在Update()每次调用完一次后,紧跟着调用一次
OnDisable():与OnEnable()相反,组件未激活时刻调用
OnDestroy():被销毁后调用一次
向量,坐标,旋转,缩放–Vector3的使用
Vector3 v= newVector(1,1,1);
Vector3 v2 = new Vector(1,1,0);
Vector3.Angle(v,v2);//两向量夹角
Vector3.Distance(v.v2);//两向量之间的距离
Vector3.Dot(v,v2);//两向量点乘
Vector3.Cross(v,v2);//两向量叉乘
Vector3.Lerp(Vector3.zero,vector3.one,0.8f);//插值
v.magnitude;//向量的模
v.normalized;//规范化向量
旋转:欧拉角,四元数
//欧拉角创建
Vector3 roate = new Vector3(0,30,0);
//四元数创建
Quaternion quaternion = Quaternion.identity;//创建没有旋转初始值为(0,0,0)的角度
//欧拉角转换为四元数
quaternion = Quaternion.Euler(ratate);
//四元数转换为欧拉角
rotate = quaternion.eulerAngles;
//看向一个物体穿件四元数
quaternion = Quaternion.LookRotation(New Vector3(0,0,0));
调试
//普通调试
Debug.Log("test");
//提醒,警告
Debug.LogWarning("text2");
//错误
Debug.LogError("text3");
绘制线条
//绘制线段
Debug.DrawLine(new Vector3(1,0,0),new Vector3(1,1,0),Color.blue);
//绘制射线
Debug.DrawRay(new Vector3(1,0,0),new Vector3(1,1,0),Color.red);
挂载的脚本拿到游戏物体
//获取游戏对象
GameObject go = this.gameObject;
//获取对象名称
Debug.Log(gameObject.name);
//获取对象的标签
Debug.Log(gameObject.tag);
//获取对象的图层
Debug.Log(gameObject.layer);
public GameObject Cube;//游戏中的物体
//获取预设体
public GameObject Prefab;
//当前真正激活的状态
Debug.Log(Cube.activeInHieraachy);
//当前自身激活的状态
Debug.Log(Cube.activeSelf);
//获取,添加组件
//获取Transform组件
Transform trans = this.transform;
Debug.Log(transform.position);
//获取其他组件
BoxCollider bc = GetComponent<要获取组件的泛型>();
//获取当前物体的子物体身上的某个组件
GetComponentInChildren<CapsuleCollider>(bc);
//获取当前物体的父物体身上的某个组件
GetComponentInParent<BoxCollider>();
//添加一个组件
Cube.AddComponent<AudioSource>();
//通过物体名字在本物体的脚本内获取其他物体
GameObject text = GameObject.Find("物体名称");
//通过物体标签在本物体的脚本内获取其他物体
text = GameObject.FindWithTag("物体标签");
//设置物体激活状态
text.SetActive(false);
//通过预设体实例化一个游戏物体
Instantiate(Prefab);
//设定预设体的父物体
Instantiate(Prefab,transform);//将实例化的物体作为本物体的子物体
//设定预设体的初始位置
Instantiate(Prefab,Vector3.zero,Quaternion.identity);//初始位置为世界的中心并且没有旋转
//销毁物体
Destory(go);
//游戏开始到现在所花的时间
Debug.Log(Time.time);
//时间缩放值
Debug.Log(Time.timeScale);
//固定时间间隔
Debug.Log(Time.fixedDeltaTime);
//Application类的属性与方法
//游戏数据文件夹路径(只读,加密文件)
Debug.Log(Application.dataPath);
//持久化文件夹路径(可写)
Debug.Log(Application.persistentDataPath);
//StreamingAssets文件夹路径(只读,配置文件)
Debug.Log(Application.StreamingAssetsPath);
//临时文件夹
Debug.Log(Application.temporaryCachepath);
//控制游戏是否早后台运行
Debug.Log(Application.runInBackground);
//打开URL
Application.OpenURL("输入URL地址");
//退出游戏
Application.Quit();
用脚本实现场景切换,场景加载,场景卸载
//通过场景名称,跳转,加载场景
SceneManager.LoadScence("目标场景名称");
//获取当前场景
Scence scence = ScenceManage.GetActiveScence();
Debug,Log(scene.name);//输出场景名字
//场景是否已加载
Debug.Log(scene.isLoaded);
//场景路径
Debug.Log(scene.path);
//场景索引
Debug.Log(scene.buildIndex);
//返回当前场景的所有游戏物体
GameObject[] gos = scene.GetRootGameObject();
Debug.Log(gos.length);
//场景管理类
//当前已加载场景的数量
Debug.Log(SceneManager.sceneCount);
//创建新场景
Scene newScene = SceneManager.CreateScene("新场景名称");
//卸载场景(异步卸载)
SceneManager.UnloadSceneAsync(newScene);
//加载场景方法2
SceneManager.LoadScene("场景名称",LoadSceneMode.Additive);//此方法会将加载出的场景内容与当前的场景内容叠加进行展示
场景的异步加载
在实现场景异步加载前应该将需要加载的场景加进Build中
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class SceneChange : MonoBehaviour
{
AsyncOperation operation;
void Start()
{
//调用场景切换方法
StartCoroutine(loadScene());
}
//协程方法用来异步加载场景
IEnumerator loadScene() {
operation = SceneManager.LoadSceneAsync(1);//异步切换场景
//加载完之后不要自动跳转
operation.allowSceneActivation = false;
yield return operation;
}
float timer = 0;
void Update()
{
//输出加载进度 进度范围0--0.9
Debug.Log(operation.progress);
//设置时间变量限制跳转时间
timer += Time.deltaTime;
//如果等待6秒就自动跳转
if(timer > 6){
operation.allowSceneActivation = true;
}
}
}
组件之间的父子级关系是靠transform来决定维系的
我们可以通过transform来控制物体的位置,旋转,缩放和父子级
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SphereText : MonoBehaviour
{
void Start()
{
//获取物体位置
Debug.Log(transform.position);//本物体相对于父物体的相对位置
Debug.Log(transform.localPosition);//本物体在场景之中的位置
//获取旋转
Debug.Log(transform.rotation);//获取物体相对于父物体的旋转
Debug.Log(transform.localRotation);//获取物体在场景中的旋转
Debug.Log(transform.eulerAngles);//获取物体相对于父物体的四元数
Debug.Log(transform.localEulerAngles);//获取在场景中的四元数
//获取缩放
Debug.Log(transform.localScale);
//向量 三个坐标轴的方向向量
Debug.Log(transform.forward);
Debug.Log(transform.right);
Debug.Log(transform.up);
//父子关系
GameObject gao = transform.parent.gameObject;//获取父物体
//获取子物体的数量
Debug.Log(transform.childCount);
//解除与子物体的父子关系
transform.DetachChildren();
//获取子物体
Transform trans = transform.Find("Child");//按照名字进行查找
trans = transform.GetChild(0);//按照编号进行查找
//判断一个物体是不是另一个物体的子物体
bool res = trans.IsChildOf(transform);
Debug.Log(res);
//设置为父物体
trans.SetParent(transform);
}
void Update()
{
//让本物体时时刻刻都看向固定位置
transform.LookAt(Vector3.zero);
//旋转
transform.Rotate(Vector3.up,1);
//绕某个物体旋转
transform.RotateAround(Vector3.zero,Vector3.up,1);
//移动
transform.Translate(Vector3.forward*0.1f);
}
}
键盘鼠标操作
因为监听鼠标键盘操作需要实时监听,所以需要将此操作放在Update()方法里
扫描二维码关注公众号,回复:
14743176 查看本文章
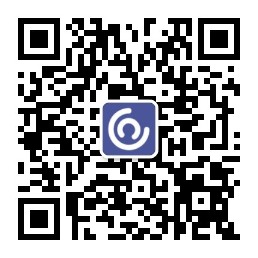
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class keyboard : MonoBehaviour
{
void Start()
{
}
void Update()
{
//监听鼠标 0左键 1右键 2滚轮
//按下鼠标键不放时触发
if (Input.GetMouseButton(0)) {
Debug.Log("持续按下鼠标左键");
}
//按下鼠标时触发
if (Input.GetMouseButtonDown(0)) {
Debug.Log("按下鼠标左键");
}
//松开鼠标键时触发
if (Input.GetMouseButtonUp(0)) {
Debug.Log("松开鼠标左键");
}
//监听键盘
//按下键盘不松时触发
if (Input.GetKey(KeyCode.A)) {
Debug.Log("持续按下A键");
}
//按下A键时触发
if (Input.GetKeyDown(KeyCode.A)) {
Debug.Log("按下A键");
}
if(Input.GetKeyUp("a")){
Debug.Log("松开A键");
}
}
}