Drawable表示一种可绘制的内容,可以由图片或者颜色组成.Android下的Drawable有BitmapDrawable、GradientDrawable、LayerDrawable等等
1.BitmapDrawable
它表示一张图片,我们可以直接将图片放在drawable目录下,该图片就可以直接作为drawable引用,当然也可以用xml的方式来描述一张图片.
<?xml version="1.0" encoding="utf-8"?>
<bitmap
xmlns:android="http://schemas.android.com/apk/res/android"
//图片的路径,必须提供,引用mipmap下的图片是没有提示的,手动补全是可以使用的,不知道为什么
android:src="@mipmap/miku"
//抗锯齿,使图片更平滑,开不开都没关系,说实话看不出来差别
android:antialias="true"
//抖动效果,在低质量的设备上提供较好的显示效果,推荐true,
android:dither="true"
//图片的透明度,取值0.0f~1.0f,0完全透明,1不透明
android:alpha="1"
//平铺模式,clamp:底部像素延伸,mirror:水平和竖直镜像平铺,repeat:水平和竖直方向平铺
//注意:如果设置为ImageView的src属性,则不会出现这些效果,做View的背景才能看到
android:tileMode="clamp"
//开启过滤,当图片被拉伸或压缩的时候能保持较好的效果,推荐true
android:filter="true"
//对齐方式,当图片的宽高小于容器View的宽高时从哪个方向开始显示,参考TextView的文字对齐方式
android:gravity="top"
//色调,99.99%的情况都不需要设置,需要配合tintMode一起使用,不然设置的颜色会挡住图片
android:tint="#39c5bb"
//色调模式,取值:multiply、src_over、screen等等,不少取值会遮盖住图片
android:tintMode="src_over">
</bitmap>
tileMode = “clamp”
tileMode = “repeat”
tileMode = “mirror”
tint = “#39c5bb” + tintMode = “multiply”
2.ShapeDrawable
ShapeDrawable是指在xml文件中的shape标签,实际对应的类是 GradientDrawable
,它表示一个图形.
跟BitmapDrawable一致的属性就不详细说明了.
<?xml version="1.0" encoding="utf-8"?>
<shape
//图形的基本形状,默认是矩形,取值: rectangle、oval,line,ring
android:shape="rectangle"
xmlns:android="http://schemas.android.com/apk/res/android"
>
//描边,边宽度,边颜色,line和ring必须设置这个,否则无法正常显示
//dashWidth需要配合dashGap一起使用,两个值都不为0,会显示虚线效果
<stroke android:width="5dp" android:color="#39c5bb"/>
//填充色,决定了形状的整体颜色
<solid android:color="#000"/>
//指定形状的宽高,未指定size,getIntrinsicWidth()等方法返回-1
<size android:width="100dp" android:height="100dp"/>
//可以实现圆角效果的半径,对于矩形,则四个角都会改变,也可以单独设置两个角的圆角效果
//bottomLeftRadius、bottomRightRadius...
<corners android:radius="10dp"/>
//渐变色,该节点和solid冲突,只能有一个,具体效果见下图
//angle:会影响渐变的方向,该值需要是45的倍数
//centerColor:渐变的中间色
//startColor:渐变的起始色
//endColor:渐变的结束色
//type:渐变的类型,取值:sweep(扫描渐变,想象一下雷达)、radial(径向渐变)、linear(线性渐变,默认)
//gradinetRaduis:只有type = "radial"有效,渐变半径
//useLevel:一般false,作为StateListDrawable为true
//centerY:渐变中心点的Y坐标,会影响渐变效果
//centerX:渐变中心点的X坐标,会影响渐变效果
<gradient android:angle="45" android:centerColor="#f00" android:startColor="#fff" android:endColor="#000"/>
</shape>
3.LayerDrawable
它表示一个拥有层次化的Drawable集合,对应标签是layer-list.它可以包含多个drawable,通过LayerDrawable可以实现一些叠加的效果.
比如:
对于上图的效果要设置给ImageView的src属性才能看到效果.
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
//item 表示一个drawable对象,也可以通过android:drawable属性来引用一个现有drawable对象
//
<item>
//可以设置一个drawable的旋转角度,pivotY,pivotX旋转的偏移量
<rotate android:fromDegrees="90">
<shape android:shape="rectangle">
<solid android:color="#ff0000"/>
<corners android:radius="10dp"/>
//这里设置的width和height对于View来说是没有任何意义的,它会按照View的大小来自适应
<size android:height="20dp" android:width="300dp"/>
</shape>
</rotate>
</item>
<item>
<rotate android:fromDegrees="45">
<shape android:shape="rectangle">
<solid android:color="#00ff00"/>
<corners android:radius="10dp"/>
<size android:height="20dp" android:width="300dp"/>
</shape>
</rotate>
</item>
<item>
<rotate android:fromDegrees="135">
<shape android:shape="rectangle">
<solid android:color="#0000ff"/>
<corners android:radius="10dp"/>
<size android:height="20dp" android:width="300dp"/>
</shape>
</rotate>
</item>
<item>
<rotate android:fromDegrees="180">
<shape android:shape="rectangle">
<solid android:color="#39c5bb"/>
<corners android:radius="10dp"/>
<size android:height="20dp" android:width="300dp"/>
</shape>
</rotate>
</item>
</layer-list>
比较常用的属性有android:left、android:top、android:right、android:bottom,分别表示drawable相对于View的上下左右的偏移量.
最后
对于很多初中级Android工程师而言,想要提升技能,往往是自己摸索成长。而不成体系的学习效果低效漫长且无助。时间久了,付出巨大的时间成本和努力,没有看到应有的效果,会气馁是再正常不过的。
所以学习一定要找到最适合自己的方式,有一个思路方法,不然不止浪费时间,更可能把未来发展都一起耽误了。
如果你是卡在缺少学习资源的瓶颈上,那么刚刚好我能帮到你。以上知识笔记全部免费分享,如有需要获取知识笔记的朋友,可以点击下方二维码费领取。
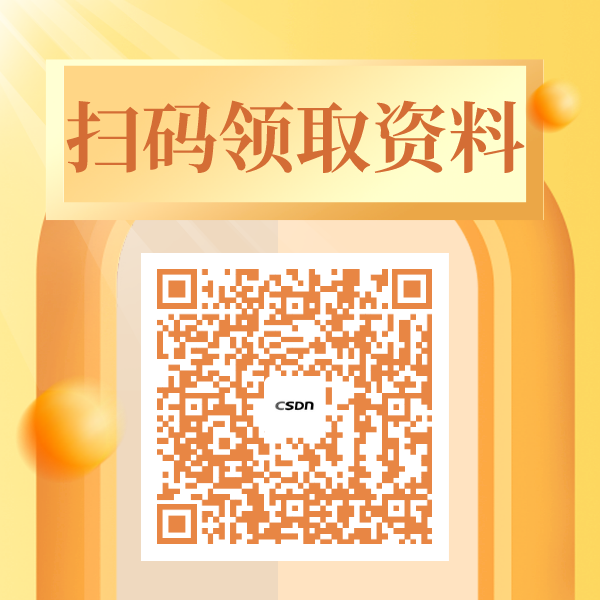