1. 介绍
Huggingface 的 transformers
库是 NLP 领域中一个重要的开源工具。与 scikit-learn
类似,Huggingface 提供了大量内置的功能和模型。其模块化设计允许用户轻松加载预训练模型,并根据需要进行微调。这篇文章将带你探索 Huggingface 的核心功能之一——pipeline
,并学习如何使用它进行情感分析、文本生成、翻译、问答等任务。
在开始之前,请确保已安装 Huggingface 所需的依赖:
pip install datasets evaluate transformers sentencepiece
2. Pipeline 基础
Pipeline 是 Huggingface 中最基础的对象,它将模型与必要的预处理和后处理步骤连接起来,使我们可以直接输入文本并获得有意义的结果。
2.1 创建情感分析 Pipeline
from transformers import pipeline
# 加载情感分析模型
classifier = pipeline("sentiment-analysis", model="distilbert-base-uncased-finetuned-sst-2-english")
print(classifier("I've been waiting for a HuggingFace course my whole life."))
你甚至可以传递多条句子进行批量分析:
classifier([
"I've been waiting for a HuggingFace course my whole life.",
"I hate this so much!"
])
Pipeline 处理过程主要分为三步:
- 预处理:将文本转换为模型可理解的格式。
- 模型推理:将预处理后的输入传递给模型。
- 后处理:将模型输出转换为可解释的结果。
3. 可用的 Pipeline 类型
目前可用的 pipeline 包括:
- 特征提取(feature-extraction)
- 填充掩码(fill-mask)
- 命名实体识别(ner)
- 问答(question-answering)
- 情感分析(sentiment-analysis)
- 文本生成(text-generation)
- 文本摘要(summarization)
- 翻译(translation)
- 零样本分类(zero-shot-classification)
3.1 零样本分类
零样本分类不需要对模型进行微调,能够直接对给定标签进行分类:
classifier = pipeline("zero-shot-classification", model="facebook/bart-large-mnli")
print(classifier(
"This is a course about the Transformers library",
candidate_labels=["education", "politics", "business"]
))
3.2 文本生成
通过输入提示,模型会自动完成剩余部分的生成:
generator = pipeline("text-generation", model="gpt2", max_length=30, pad_token_id=0)
print(generator("In this course, we will teach you how to"))
3.3 掩码填充
掩码填充是语言模型的重要任务之一:
unmasker = pipeline("fill-mask", model="distilroberta-base")
print(unmasker("This course will teach you all about <mask> models.", top_k=2))
3.4 命名实体识别
Huggingface 也支持多样化的 NER 模型:
ner = pipeline("ner", aggregation_strategy="simple", model="dbmdz/bert-large-cased-finetuned-conll03-english")
print(ner("My name is Sylvain and I work at Hugging Face in Brooklyn."))
3.5 问答系统
基于模型的问答功能如下:
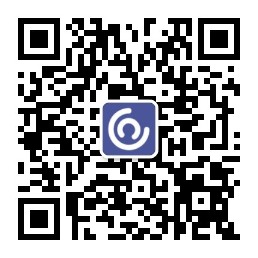
question_answerer = pipeline("question-answering", model="distilbert-base-cased-distilled-squad")
print(question_answerer(
question="Where do I work?",
context="My name is Sylvain and I work at Hugging Face in Brooklyn."
))
3.6 文本摘要
使用预训练模型生成文本摘要:
summarizer = pipeline("summarization", model="sshleifer/distilbart-cnn-12-6", max_length=100)
print(summarizer(
"""
America has changed dramatically during recent years. Not only has the number of
graduates in traditional engineering disciplines such as mechanical, civil,
electrical, chemical, and aeronautical engineering declined, but in most of
the premier American universities engineering curricula now concentrate on
and encourage largely the study of engineering science...
"""
))
3.7 翻译
translator = pipeline("translation", model="Helsinki-NLP/opus-mt-fr-en")
print(translator("Ce cours est produit par Hugging Face."))
4. Transformers 模型简介
Huggingface 的 transformer 模型主要分为三类:
- BERT-like(编码器模型):适合分类、NER 等任务。
- GPT-like(解码器模型):适合生成任务。
- BART/T5-like(编码器-解码器模型):适合翻译、摘要等任务。
这些模型在大量文本上进行了预训练,可以通过微调进一步提升在特定任务上的表现。
5. 模型中的偏见与局限
由于模型基于真实世界的数据进行训练,因此可能会带有一些潜在的偏见。例如:
unmasker = pipeline("fill-mask", model="distilroberta-base")
print(unmasker("This man works as a <mask>."))
print(unmasker("This woman works as a <mask>."))
6. Pipeline 的背后
Pipeline 连接了预处理、模型推理和后处理三部分。以下是情感分析的具体实现:
from transformers import AutoTokenizer, AutoModelForSequenceClassification
checkpoint = "distilbert-base-uncased-finetuned-sst-2-english"
tokenizer = AutoTokenizer.from_pretrained(checkpoint)
model = AutoModelForSequenceClassification.from_pretrained(checkpoint)
inputs = tokenizer(["I love this!", "I hate this!"], padding=True, truncation=True, return_tensors="pt")
outputs = model(**inputs)
print(outputs.logits)
通过 SoftMax
将 logits 转换为概率:
import torch
predictions = torch.nn.functional.softmax(outputs.logits, dim=-1)
print(predictions)
结语
在本篇文章中,我们学习了如何使用 Huggingface 的 pipeline
执行多种 NLP 任务,包括情感分析、文本生成、问答和翻译等。Huggingface 的模型不仅易于使用,还能通过简单的代码实现复杂的自然语言处理功能。
在接下来的文章中,我们将深入探讨如何使用 Huggingface 的自定义分词器和数据集加载功能。这些工具将帮助我们进一步优化模型性能,并为更复杂的 NLP 项目打下坚实的基础。
敬请期待《基于Python的自然语言处理系列(34):Huggingface 自定义分词器》!
如果你觉得这篇博文对你有帮助,请点赞、收藏、关注我,并且可以打赏支持我!
欢迎关注我的后续博文,我将分享更多关于人工智能、自然语言处理和计算机视觉的精彩内容。
谢谢大家的支持!