本文通过一个hello world简单示例演示,如何基于Spring Boot集成Spring AI Alibaba开源框架,快速集成调用阿里云的百炼大模型AI服务。
1、概念介绍
Spring AI Alibaba 项目
Spring AI Alibaba 开源项目基于 Spring AI 构建,是阿里云通义系列模型及服务在 Java AI 应用开发领域的最佳实践,提供高层次的 AI API 抽象与云原生基础设施集成方案,帮助开发者快速构建 AI 应用。 Spring AI Alibaba 作为开发 AI 应用程序的基础框架,定义了以下抽象概念与 API,并提供了 API 与通义系列模型的适配。
-
Spring AI 项目
Spring AI 项目由 Spring 官方开源并维护的 AI 应用开发框架,该项目目标是简化包含人工智能(AI)功能的应用程序的开发,避免不必要的复杂性。该项目从著名的 Python 项目(例如 LangChain 和 LlamaIndex)中汲取灵感,但 Spring AI 并非这些项目的直接移植,该项目的成立基于这样的信念:下一波生成式 AI 应用将不仅面向 Python 开发人员,还将遍及多种编程语言。从本质上讲,Spring AI 解决了 AI 集成的基本挑战:Connecting your enterprise Data and APIs with the AI Models。
2、使用Spring AI Alibaba前提条件
- JDK为17以上版本,本人使用的jdk21版本;
- SpringBoot版本为3.x以上,本项目使用的是SpringBoot 3.3.3版本;
- 开通阿里大模型服务(目前是免费6个月),获取 API-KEY,后面代码里要使用。具体操作,请参考阿里云大模型服务平台百炼:如何获取API Key_大模型服务平台百炼(Model Studio)-阿里云帮助中心
3、创建SpringBoot工程
通过IDEA开发工具,创建一个普通的Java工程,注意JDK版本至少是17以上,我这里使用的是jdk21版本。
4、配置pom.xml文件
工程创建完成后,在pom.xml里添加依赖。
首先,需要在项目中添加 spring-boot-starter-parent声明,指定springboot框架的版本号:
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.3.3</version>
<relativePath/>
</parent>
在dependencies标签中引入spring-boot-starter-web和spring-ai-alibaba-starter依赖:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud.ai</groupId>
<artifactId>spring-ai-alibaba-starter</artifactId>
<version>1.0.0-M3.2</version>
</dependency>
</dependencies>
由于 spring-ai 相关依赖包还没有发布到中央仓库,如出现 spring-ai-core 等相关依赖解析问题,请在您项目的 pom.xml 依赖中加入如下仓库配置。
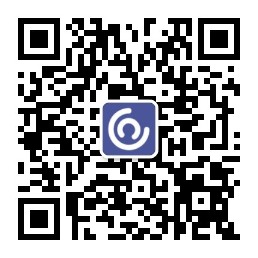
<repositories>
<repository>
<id>spring-milestones</id>
<name>Spring Milestones</name>
<url>https://repo.spring.io/milestone</url>
<snapshots>
<enabled>false</enabled>
</snapshots>
</repository>
</repositories>
最后pom.xml文件完整内容如下:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.yuncheng</groupId>
<artifactId>spring-ai-helloworld</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>21</maven.compiler.source>
<maven.compiler.target>21</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.3.3</version>
<relativePath/>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud.ai</groupId>
<artifactId>spring-ai-alibaba-starter</artifactId>
<version>1.0.0-M3.2</version>
</dependency>
</dependencies>
<repositories>
<repository>
<id>spring-milestones</id>
<name>Spring Milestones</name>
<url>https://repo.spring.io/milestone</url>
<snapshots>
<enabled>false</enabled>
</snapshots>
</repository>
</repositories>
</project>
5、配置yml文件
在工程的resources目录下的application-dev.yml文件里增加如下配置:
注意:api-key要替换成自己从阿里百炼平台申请的key
server:
port: 8080
spring:
application:
name: spring-ai-helloworld
ai:
dashscope:
api-key: sk-b90ad31bb3eb4a158124928354f39dc5
6、创建ChatController
创建一个普通 Controller Bean类, 注入 ChatClient 实例,这样你的 Bean 就具备与 AI 大模型智能对话的能力了。本示例简单演示了普通的文本输出对话和流输出对话。
package com.yuncheng;
import com.alibaba.cloud.ai.dashscope.chat.DashScopeChatOptions;
import jakarta.servlet.http.HttpServletResponse;
import reactor.core.publisher.Flux;
import org.springframework.ai.chat.client.ChatClient;
import org.springframework.ai.chat.model.ChatModel;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/ai")
public class ChatClientController {
private final ChatClient chatClient;
private final ChatModel chatModel;
public ChatClientController(ChatModel chatModel) {
this.chatModel = chatModel;
// 构造时,可以设置 ChatClient 的参数
this.chatClient = ChatClient.builder(chatModel)
.defaultOptions(
DashScopeChatOptions.builder()
.withTopP(0.7)
.build()
)
.build();
}
/**
* ChatClient 简单调用
*/
@GetMapping("/simple/chat")
public String simpleChat(String input) {
return chatClient.prompt().user(input).call().content();
}
/**
* ChatClient 流式调用
*/
@GetMapping("/stream/chat")
public Flux<String> streamChat(HttpServletResponse response,String input) {
response.setCharacterEncoding("UTF-8");
return chatClient.prompt().user(input).stream().content();
}
}
可以通过设置属性 spring.ai.chat.client.enabled=true来自动装配ChatClient.Builder bean,但是,如果需要多个聊天模型一起使用,需要以编程方式创建 ChatClient.Builder,这样可以为每个聊天模型创建一个实例 ChatModel,这里使用的是编程方式创建ChatClient。
7、创建Application启动类
package com.yuncheng;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ConfigurableApplicationContext;
import org.springframework.core.env.Environment;
@SpringBootApplication
public class AiApplication {
public static void main(String[] args) {
ConfigurableApplicationContext application = SpringApplication.run(AiApplication.class, args);
Environment env = application.getEnvironment();
String port = env.getProperty("server.port");
System.out.println("AiApplication启动成功,服务端口为:" + port);
}
}
8、启动Springboot工程并测试
工程启动成功后,可以向阿里云AI大模型提问了。
把下面地址输入到浏览器里,答案以文本方式输出:
http://localhost:8080/ai/simple/chat?input=给我讲一个关于西游记的小故事
把下面地址输入到浏览器里,答案以流方式逐步输出:
http://localhost:8080/ai/stream/chat?input=给我讲一个关于西游记的小故事
9、总结
本文通过一个经典的“Hello World”示例,初步地验证了基于Spring Boot集成Spring AI Alibaba开源框架的可行性和简便性。该实验不仅展示了如何通过编程方式灵活定义`ChatClient`,还深入探讨了利用Spring AI Alibaba框架调用阿里巴巴AI大模型API接口的具体实现路径,从而支持普通文本问答和流式问答两种交互模式。这一集成不仅为Java开发者提供了一种高效、便捷的方式去探索和利用最新的AI技术,同时也为未来更复杂的AI应用场景奠定了坚实的技术基础。
1)环境搭建:
- 选择Spring Boot作为基础开发框架,因其具备快速启动、自动配置等特性,能够显著简化项目初始化过程。
- 引入Spring AI Alibaba依赖库,确保应用程序可以无缝接入阿里巴巴提供的先进AI能力。
2)ChatClient定义:
- 采用面向对象编程方法,设计并实现了ChatClient类,该类封装了与AI大模型API交互所需的所有逻辑,包括但不限于请求构造、响应解析等功能。
- ChatClient的设计遵循了高内聚低耦合的原则,使得其既易于维护又便于扩展,为后续功能增强提供了良好的架构基础。
3)API接口调用:
- 普通文本问答:通过构建标准HTTP POST请求,向阿里巴巴AI大模型API发送预处理后的用户输入文本,并接收返回的结果。此过程验证了从简单的文本查询到复杂语义理解的能力。
- 流式问答:引入Flux或类似技术实现双向通信,允许客户端实时接收来自服务器端的流式数据更新。这不仅提升了用户体验,还展示了在处理连续对话或长时间任务时的优势。
4)结果分析:
- 实验结果显示,通过Spring Boot集成Spring AI Alibaba框架,开发者可以在保持原有应用结构不变的情况下,轻松添加强大的AI功能。
- 测试过程中,无论是普通文本问答还是流式问答,系统的响应速度和准确性均达到了预期目标,证明了该集成方案的有效性和稳定性。