一:springboot热启动
1:热启动插件依赖
1 2 3 4 |
|
该插件功能:是boot的一个热部署工具,当我们修改了(类、属性文件、页面等)时,会自动重新启动应用(由于其采用的双类加载器机制,这个启动会非常快,比手动重启快很多倍)
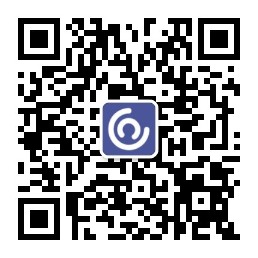
2:启动入口程序的方式必须是runas----->springboot app
注意:如果在pom文件添加依赖建议重新启动工程
二:自定义属性配置
定义:在application.properties属性配置文件中定义属性名和属性值
1 |
|
取值:在控制器中取值
1 2 |
|
三:默认属性配置
Springboot有很多默认配置参考springboot文档。
比如:编码对中文支持很友好,统一utf-8
1 2 3 4 |
|
比如:tomcat端口号
1 |
|
比如thymeleaf模板引擎的默认配置
四:修改默认配置
1 2 3 |
|
五:通过属性配置文件配置随机数
1:重新新建一个random.properties属性配置文件,如下图:
2:配置随机数信息如下:
1 2 3 4 5 6 7 8 9 10 |
|
3:将属性注入到一个Pojo实体类,这种注法,你发现并没有使用@Value注解哦,怎么做到的呢?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
|
4:Springboot的单元测试随机数
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
|
无需导入相关jar因为在新建spring boot 项目时会自动引入snakeyaml,从而自动实现对yaml的支持
举例子:
environments: dev: url: http://dev.bar.com name: Developer Setup prod: url: http://foo.bar.com name: My Cool App |
Would be transformed into these properties:
environments.dev.url=http://dev.bar.com environments.dev.name=Developer Setup environments.prod.url=http://foo.bar.com environments.prod.name=My Cool Ap |
注意:
1:一定要注意冒号后一定要加空格,要不然就无法生效
2:大小写敏感
3:使用缩进表示层级关系
4:缩进时不允许使用Tab键,只允许使用空格。
6:缩进的空格数目不重要,只要相同层级的元素左侧对齐即可
Yaml高级用法:http://samchu.logdown.com/posts/290211-spring-boot-yaml-uses
命令:java -jar xxx.jar --server.port=8888 通过使用–-server.port属性来设置xxx.jar应用的端口为8888。 java -jar xxx.jar --server.port=8888命令,等价于我们在application.properties中添加属性server.port=8888 |