Given a pair of positive integers, for example, 6 and 110, can this equation 6 = 110 be true? The answer is “yes”, if 6 is a decimal number and 110 is a binary number.
Now for any pair of positive integers N1 and N2, your task is to find the radix of one number while that of the other is given.
Input Specification:
Each input file contains one test case. Each case occupies a line which contains 4 positive integers:\ N1 N2 tag radix\ Here N1 and N2 each has no more than 10 digits. A digit is less than its radix and is chosen from the set {0-9, a-z} where 0-9 represent the decimal numbers 0-9, and a-z represent the decimal numbers 10-35. The last number “radix” is the radix of N1 if “tag” is 1, or of N2 if “tag” is 2.
Output Specification:
For each test case, print in one line the radix of the other number so that the equation N1 = N2 is true. If the equation is impossible, print “Impossible”. If the solution is not unique, output the smallest possible radix.
Sample Input 1:
6 110 1 10
Sample Output 1:
2
Sample Input 2:
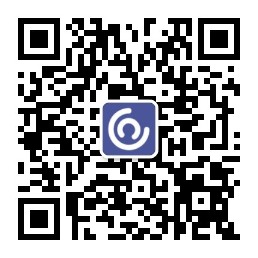
1 ab 1 2
Sample Output 2:
Impossible
利用二分进制的思路很巧妙,不过要注意数据可能会很大,尽量都用LL;进制转换的时候要注意判断溢出,否则只有15分。
疑问:为什么进制的上界是max(low,num1)+1???
#include<cstdio>
#include<cstring>
#include<algorithm>
using namespace std;
typedef long long LL;
LL map[256];
void init() { //map数组将字符转换为数字之用
for (char c = '0'; c <= '9'; c++)
map[c] = c - '0';
for (char c = 'a'; c <= 'z'; c++)
map[c] = c - 'a' + 10;
}
LL ConvertNum(char N[],LL radix ) {
LL ans = 0;
int len = strlen(N);
for (int i = 0; i < len; i++) {
ans = ans * radix + map[N[i]];
}
if (ans < 0) return -1;//小于0,溢出
else return ans;
}
int BinarySearch(LL num1,LL low,LL high,char N[] ) {
LL num2, mid;
while (low <= high) {
mid = (low + high) / 2;
num2 = ConvertNum(N, mid);
if (num1 == num2) return mid;
if (num2 < 0 || num2>num1) high = mid - 1;//题目给的数据,num1不会溢出,num2会溢出
else low = mid + 1;
}
return -1;
}
int FindMaxDigit(char N[]) {
int len = strlen(N), MaxDigit = -1;
for (int i = 0; i < len; i++) {
if (map[N[i]] > MaxDigit) MaxDigit = map[N[i]];
}
return MaxDigit + 1;//进制的下界
}
int main() {
char N1[15], N2[15];
int tag, radix;
scanf("%s %s %d %d", N1, N2, &tag, &radix);
init();
if (tag == 2) swap(N1, N2);
LL num1 = ConvertNum(N1,radix);
LL low = FindMaxDigit(N2);
LL high = max(low, num1) + 1;
int ans = BinarySearch(num1, low, high, N2);
if (ans > 0) printf("%lld\n", ans);
else printf("Impossible\n");
return 0;
}