1010 Radix (25 point(s))
Given a pair of positive integers, for example, 6 and 110, can this equation 6 = 110 be true? The answer is yes
, if 6 is a decimal number and 110 is a binary number.
Now for any pair of positive integers N1 and N2, your task is to find the radix of one number while that of the other is given.
Input Specification:
Each input file contains one test case. Each case occupies a line which contains 4 positive integers:
N1 N2 tag radix
Here N1
and N2
each has no more than 10 digits. A digit is less than its radix and is chosen from the set { 0-9, a
-z
} where 0-9 represent the decimal numbers 0-9, and a
-z
represent the decimal numbers 10-35. The last number radix
is the radix of N1
if tag
is 1, or of N2
if tag
is 2.
Output Specification:
For each test case, print in one line the radix of the other number so that the equation N1
= N2
is true. If the equation is impossible, print Impossible
. If the solution is not unique, output the smallest possible radix.
Sample Input 1:
6 110 1 10
Sample Output 1:
2
Sample Input 2:
1 ab 1 2
Sample Output 2:
Impossible
题目大意
给定两个数字和一个标签以及对应一个数字的基数
给出另一个数字的基数,如果不存在,则返回 Impossible
如果tag==1 表示的是对应的题目给的基数和第一个数字对应
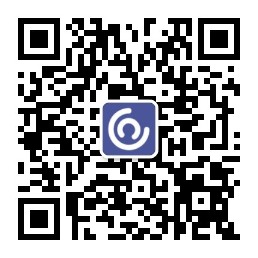
反之和第二个数字对应
1.注意点,对应的所求的基数范围并不是到36为止,可能是无限大,想象一下,完全是有可能的
2.对应的用string 去接N1,N2 ,然后再进行数值的计算
3.将所求的基数限定在所求的位数+1,作为最小下限,同时将另一个确定的数字作为最大的基数来进行二分搜索,减少复杂度
4.中间的计算可能会有溢出,所以可以通过先判断是否小于0来进行判断是否溢出了,这里要先做判断,可以结合代码,在二分法的下面有一块注释,读着感觉差不多,但是实际上下面的不能全部通过.(或者换成unsigned long long 也能解决)
#include<iostream>
#include<math.h>
using namespace std;
long long getNum(string input ,long long radix){
long long sum=0;
long long index =0;
for(long long i=input.length()-1;i>=0;i--){
long long temp =0;
if('0'<=input[i]&&input[i]<='9'){
temp = (input[i] - '0');
}else{
temp = (input[i] - 'a')+10;
}
sum+= temp*pow(radix,index++);
}
return sum;
}
long long getMAX_radix(string input){
long long max=0;
for(unsigned long long i=0;i<input.length();i++){
long long temp =0;
if('0'<=input[i]&&input[i]<='9'){
temp = (input[i] - '0');
}else{
temp = (input[i] - 'a')+10;
}
if(temp>max){
max = temp;
}
}
return max+1;
}
long long find_radix(string str,long long num){
//long long a1 = getNum(str,num);
//cout<<"a1:"<<a1<<endl;
long long left = getMAX_radix(str);
long long right = max(num,left);
long long the_final =-1;
while(left<=right){
long long mid = (left+right)/2;
long long result = getNum(str,mid);
//cout<<"result:"<<result<<endl;
if(result<0||result>num){
right = mid -1;
}else if(result<num){
left = mid+1;
}else{
the_final = mid;
break;
}
// be careful about this codes block
// if(result == num){
// the_final = mid;
// break;
// }else if(result<num){
// left = mid+1;
// }else{
// right = mid -1;
// }
}
return the_final;
}
int main(){
string n1,n2;
long long tag,radix;
cin>>n1>>n2>>tag>>radix;
long long num = (tag==1)?getNum(n1,radix):getNum(n2,radix);
long long the_final = (tag==1)?find_radix(n2,num):find_radix(n1,num);
if(the_final==-1){
cout<<"Impossible"<<endl;
}else{
cout<<the_final<<endl;
}
//cout<<"sds:"<<'a' - 'a'+10<<endl;
return 0;
}
radix
- n. 根;[数] 基数
- n. (Radix)人名;(法、德、西)拉迪克斯;(英)雷迪克斯