虚拟摄像机开发的最佳实践和技巧
在上一节中,我们已经探讨了虚拟摄像机的基本概念和设置方法。接下来,我们将深入探讨虚拟摄像机开发的最佳实践和技巧,帮助开发者在Unreal Engine中实现更加流畅、自然且功能丰富的摄像机系统。
1. 摄像机平滑过渡
1.1 使用插值函数实现平滑过渡
在Unreal Engine中,使用插值函数可以帮助实现摄像机位置和旋转的平滑过渡。插值函数可以通过时间或距离来调整摄像机的移动和旋转,从而避免突兀的跳变,提升玩家的游戏体验。
1.1.1 时间插值
时间插值是最常见的平滑过渡方法之一。我们可以使用FMath::VInterpTo
和FMath::RInterpTo
函数来实现摄像机的位置和旋转插值。
// 在角色类中定义摄像机平滑过渡的函数
void AMyCharacter::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
// 目标位置和旋转
FVector TargetLocation = DesiredCameraLocation;
FRotator TargetRotation = DesiredCameraRotation;
// 使用时间插值函数进行平滑过渡
CameraComponent->SetWorldLocation(FMath::VInterpTo(CameraComponent->GetWorldLocation(), TargetLocation, DeltaTime, 10.0f));
CameraComponent->SetWorldRotation(FMath::RInterpTo(CameraComponent->GetWorldRotation(), TargetRotation, DeltaTime, 10.0f));
}
在这个例子中,DesiredCameraLocation
和DesiredCameraRotation
是摄像机的目标位置和旋转。FMath::VInterpTo
和FMath::RInterpTo
函数的第一个参数是当前值,第二个参数是目标值,第三个参数是时间步长,第四个参数是插值速度。插值速度越低,过渡越平滑。
1.1.2 距离插值
距离插值可以根据摄像机与目标之间的距离来调整过渡速度。这对于某些特定场景,如跟随角色或瞄准目标,非常有用。
// 在角色类中定义摄像机平滑过渡的函数
void AMyCharacter::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
// 目标位置
FVector TargetLocation = DesiredCameraLocation;
// 计算当前摄像机与目标位置之间的距离
float Distance = FVector::Distance(CameraComponent->GetWorldLocation(), TargetLocation);
// 使用距离插值函数进行平滑过渡
float InterpSpeed = FMath::Clamp(100.0f / Distance, 1.0f, 10.0f);
CameraComponent->SetWorldLocation(FMath::VInterpTo(CameraComponent->GetWorldLocation(), TargetLocation, DeltaTime, InterpSpeed));
}
在这个例子中,InterpSpeed
是根据摄像机与目标位置之间的距离动态调整的。FMath::Clamp
函数确保插值速度在合理范围内。
2. 摄像机跟随
2.1 基于角色的摄像机跟随
在动作游戏中,摄像机通常需要跟随角色的移动。我们可以使用Spring Arm
组件来实现这一点,该组件可以自动调整摄像机的位置和方向,使其平滑地跟随角色。
2.1.1 设置Spring Arm组件
首先,我们需要在角色蓝图中添加一个Spring Arm
组件,并将其与摄像机组件关联。
// 在角色类的构造函数中设置Spring Arm组件
AMyCharacter::AMyCharacter()
{
// 创建Spring Arm组件
SpringArmComponent = CreateDefaultSubobject<USpringArmComponent>(TEXT("SpringArmComponent"));
SpringArmComponent->SetupAttachment(RootComponent);
SpringArmComponent->TargetArmLength = 300.0f; // 设置目标长度
SpringArmComponent->SocketOffset = FVector(0.0f, 0.0f, 75.0f); // 设置偏移
SpringArmComponent->bUsePawnControlRotation = true; // 使用角色的控制旋转
// 创建摄像机组件并附着到Spring Arm
CameraComponent = CreateDefaultSubobject<UCameraComponent>(TEXT("CameraComponent"));
CameraComponent->SetupAttachment(SpringArmComponent);
}
2.1.2 动态调整Spring Arm参数
在某些情况下,我们可能需要根据游戏状态动态调整Spring Arm
的参数,以适应不同的场景需求。
// 在角色类中定义动态调整Spring Arm参数的函数
void AMyCharacter::AdjustSpringArm(float NewTargetArmLength, float NewSocketOffsetZ)
{
SpringArmComponent->TargetArmLength = NewTargetArmLength;
SpringArmComponent->SocketOffset.Z = NewSocketOffsetZ;
}
这个函数可以根据需要调整Spring Arm
的长度和偏移量,例如在角色跳跃或蹲下时。
3. 摄像机抖动
3.1 使用Camera Shake实现摄像机抖动
摄像机抖动可以增强游戏的紧张感和沉浸感。Unreal Engine提供了UCameraShake
类来实现这一点。我们可以创建自定义的摄像机抖动类,并在需要的时候触发抖动效果。
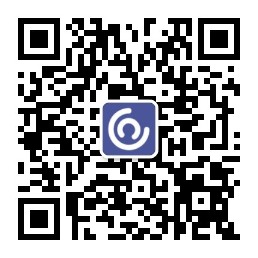
3.1.1 创建自定义摄像机抖动类
首先,我们需要创建一个新的摄像机抖动类。在Unreal Engine中,这可以通过继承UCameraShake
类来实现。
// MyCameraShake.h
#pragma once
#include "CoreMinimal.h"
#include "Camera/CameraShakeBase.h"
#include "MyCameraShake.generated.h"
UCLASS()
class MYGAME_API UMyCameraShake : public UCameraShakeBase
{
GENERATED_BODY()
public:
// 覆盖父类的 ShakeFunction
virtual void ShakeUpdateFunction(float IntensityMultiplier, float CurrentShakeFunctionTime, float ShakeCompletionTime) override;
};
// MyCameraShake.cpp
#include "MyCameraShake.h"
#include "Camera/CameraShake.h"
void UMyCameraShake::ShakeUpdateFunction(float IntensityMultiplier, float CurrentShakeFunctionTime, float ShakeCompletionTime)
{
// 定义抖动的幅度和频率
const float ShakeAmplitude = 5.0f * IntensityMultiplier;
const float ShakeFrequency = 20.0f * IntensityMultiplier;
// 计算抖动偏移
const float DeltaTime = CurrentShakeFunctionTime - LastFunctionTime;
const float Angle = ShakeFrequency * DeltaTime;
const float SinValue = FMath::Sin(Angle);
const float CosValue = FMath::Cos(Angle);
// 应用抖动偏移
AddCameraOffset(FVector(SinValue * ShakeAmplitude, CosValue * ShakeAmplitude, 0.0f));
}
在这个例子中,我们定义了一个自定义的摄像机抖动类UMyCameraShake
,并在ShakeUpdateFunction
中实现了抖动逻辑。
3.1.2 触发摄像机抖动
在游戏逻辑中,我们可以在特定事件发生时触发摄像机抖动效果。
// 在角色类中定义触发摄像机抖动的函数
void AMyCharacter::TriggerCameraShake()
{
UMyCameraShake* Shake = NewObject<UMyCameraShake>(UMyCameraShake::StaticClass());
if (Shake)
{
Controller->ClientStartCameraShake(Shake, 1.0f); // 强度为1.0
}
}
这个函数在调用时会创建一个UMyCameraShake
实例,并触发摄像机抖动效果。
4. 摄像机FoV调整
4.1 动态调整FoV
FoV(视场角)调整可以用于增强特定游戏效果,如瞄准时的缩放效果。我们可以通过设置摄像机组件的Field of View
属性来实现这一点。
4.1.1 设置FoV属性
在角色类中,我们可以定义一个函数来动态调整摄像机的FoV。
// 在角色类中定义调整FoV的函数
void AMyCharacter::AdjustCameraFoV(float NewFoV)
{
CameraComponent->SetFieldOfView(NewFoV);
}
4.1.2 使用插值函数平滑调整FoV
为了使FoV调整更加平滑,我们可以使用插值函数。
// 在角色类中定义平滑调整FoV的函数
void AMyCharacter::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
// 目标FoV
float TargetFoV = DesiredFoV;
// 使用插值函数平滑调整FoV
float CurrentFoV = CameraComponent->FieldOfView;
CameraComponent->SetFieldOfView(FMath::FInterpTo(CurrentFoV, TargetFoV, DeltaTime, 2.0f));
}
在这个例子中,DesiredFoV
是摄像机的目标FoV,FMath::FInterpTo
函数用于平滑过渡。
5. 摄像机碰撞检测
5.1 使用Trace进行碰撞检测
在某些情况下,摄像机可能会穿过场景中的障碍物。为了防止这种情况,我们可以使用Line Trace
进行碰撞检测,并根据检测结果调整摄像机的位置。
5.1.1 实现碰撞检测逻辑
首先,我们需要在角色类中实现碰撞检测逻辑。
// 在角色类中定义碰撞检测函数
void AMyCharacter::CheckCameraCollision()
{
FVector Start = CameraComponent->GetComponentLocation();
FVector End = Start + CameraComponent->GetForwardVector() * 100.0f;
FHitResult HitResult;
bool bHit = GetWorld()->LineTraceSingleByChannel(HitResult, Start, End, ECC_Visibility);
if (bHit)
{
// 如果检测到碰撞,调整摄像机位置
FVector NewCameraLocation = Start - CameraComponent->GetForwardVector() * 50.0f;
CameraComponent->SetWorldLocation(NewCameraLocation);
}
}
在这个例子中,我们使用Line Trace
检测摄像机前方是否有障碍物。如果有碰撞发生,我们调整摄像机的位置,使其远离障碍物。
5.1.2 在Tick中调用碰撞检测
为了确保每次帧都进行碰撞检测,我们可以在Tick
函数中调用CheckCameraCollision
函数。
// 在角色类的Tick函数中调用碰撞检测
void AMyCharacter::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
CheckCameraCollision();
}
6. 摄像机切换
6.1 使用Camera Manager实现摄像机切换
在某些情况下,游戏可能需要在多个摄像机之间进行切换。我们可以使用Camera Manager
来管理这些摄像机,并在需要时进行切换。
6.1.1 创建多个摄像机
首先,我们需要在角色蓝图中创建多个摄像机组件。
// 在角色类的构造函数中创建多个摄像机组件
AMyCharacter::AMyCharacter()
{
// 创建第一个摄像机组件
CameraComponent1 = CreateDefaultSubobject<UCameraComponent>(TEXT("CameraComponent1"));
CameraComponent1->SetupAttachment(SpringArmComponent);
CameraComponent1->SetRelativeLocation(FVector(0.0f, 0.0f, 150.0f));
// 创建第二个摄像机组件
CameraComponent2 = CreateDefaultSubobject<UCameraComponent>(TEXT("CameraComponent2"));
CameraComponent2->SetupAttachment(SpringArmComponent);
CameraComponent2->SetRelativeLocation(FVector(0.0f, 0.0f, 300.0f));
}
6.1.2 切换摄像机
在角色类中,我们可以定义一个函数来切换摄像机。
// 在角色类中定义切换摄像机的函数
void AMyCharacter::SwitchCamera()
{
if (CurrentCamera == CameraComponent1)
{
CurrentCamera = CameraComponent2;
}
else
{
CurrentCamera = CameraComponent1;
}
if (Controller)
{
Controller->SetViewTargetWithBlend(CurrentCamera, 0.5f); // 平滑过渡
}
}
这个函数在调用时会切换当前使用的摄像机,并使用SetViewTargetWithBlend
函数实现平滑过渡。
7. 摄像机视角限制
7.1 限制摄像机的旋转范围
在某些游戏中,摄像机的旋转范围可能需要受到限制,以避免视角过于倾斜或倒置。我们可以通过设置摄像机组件的旋转限制来实现这一点。
7.1.1 设置旋转限制
在角色类中,我们可以定义一个函数来设置摄像机的旋转限制。
// 在角色类中定义设置旋转限制的函数
void AMyCharacter::SetCameraRotationLimits(float MinPitch, float MaxPitch)
{
CameraComponent->SetWorldRotation(FRotator(FMath::Clamp(CameraComponent->GetWorldRotation().Pitch, MinPitch, MaxPitch), CameraComponent->GetWorldRotation().Yaw, CameraComponent->GetWorldRotation().Roll));
}
7.1.2 在Input处理中应用旋转限制
为了确保每次旋转都受到限制,我们可以在输入处理函数中调用SetCameraRotationLimits
函数。
// 在角色类中定义输入处理函数
void AMyCharacter::SetupPlayerInputComponent(class UInputComponent* PlayerInputComponent)
{
Super::SetupPlayerInputComponent(PlayerInputComponent);
PlayerInputComponent->BindAxis("LookUp", this, &AMyCharacter::LookUp);
PlayerInputComponent->BindAxis("Turn", this, &AMyCharacter::Turn);
}
void AMyCharacter::LookUp(float Value)
{
if (Value != 0.0f)
{
AddControllerPitchInput(Value);
SetCameraRotationLimits(-45.0f, 45.0f); // 限制俯仰角度
}
}
void AMyCharacter::Turn(float Value)
{
if (Value != 0.0f)
{
AddControllerYawInput(Value);
}
}
在这个例子中,我们限制了摄像机的俯仰角度在-45度到45度之间。
8. 摄像机后处理效果
8.1 使用Post Process Volume实现后处理效果
后处理效果可以用于增强游戏的视觉效果,如模糊、色彩校正等。我们可以通过在场景中添加Post Process Volume
并设置其参数来实现这一点。
8.1.1 创建Post Process Volume
首先,我们需要在场景中创建一个Post Process Volume
,并设置其参数。
-
在场景中添加一个
Post Process Volume
。 -
在
Post Process Volume
的详细信息面板中,添加一个Post Process Blend Weight
参数,并设置其权重。 -
添加所需的后处理效果,如
Motion Blur
、Color Grading
等。
8.1.2 动态调整后处理效果
在角色类中,我们可以定义一个函数来动态调整后处理效果的参数。
// 在角色类中定义动态调整后处理效果的函数
void AMyCharacter::AdjustPostProcessEffect(float NewMotionBlurIntensity, float NewColorGradingIntensity)
{
if (PostProcessVolume)
{
PostProcessVolume->Settings.MotionBlurAmount = NewMotionBlurIntensity;
PostProcessVolume->Settings.ColorGradingIntensity = NewColorGradingIntensity;
}
}
8.1.3 在游戏逻辑中应用后处理效果
我们可以在游戏逻辑中根据需要调用AdjustPostProcessEffect
函数,例如在角色受伤时增加模糊效果。
// 在角色类中定义受伤函数
void AMyCharacter::TakeDamage(float DamageAmount)
{
// 处理受伤逻辑
Health -= DamageAmount;
// 增加模糊效果
AdjustPostProcessEffect(0.8f, 0.5f);
// 在一段时间后恢复
GetWorld()->GetTimerManager().SetTimer(PostProcessRecoveryTimer, this, &AMyCharacter::RecoverPostProcessEffect, 2.0f, false);
}
void AMyCharacter::RecoverPostProcessEffect()
{
// 恢复后处理效果
AdjustPostProcessEffect(0.0f, 0.0f);
}
在这个例子中,当角色受伤时,我们增加了模糊效果,并在一段时间后恢复到正常状态。
9. 摄像机调试工具
9.1 使用Camera Debug工具
Unreal Engine提供了多种调试工具,可以帮助开发者调试摄像机的行为。其中,Camera Debug
工具非常有用,可以显示摄像机的位置、旋转和视场等信息。
9.1.1 启用Camera Debug
在Unreal Engine的控制台中,我们可以使用以下命令启用摄像机调试工具:
// 在游戏开始时启用Camera Debug
void AMyCharacter::BeginPlay()
{
Super::BeginPlay();
// 启用Camera Debug
GEngine->AddOnScreenDebugMessage(-1, 15.0f, FColor::Yellow, TEXT("Camera Debug Enabled"));
ShowCameraDebug();
}
void AMyCharacter::ShowCameraDebug()
{
if (GEngine)
{
GEngine->AddOnScreenDebugMessage(-1, 15.0f, FColor::Yellow, FString::Printf(TEXT("Camera Location: %s"), *CameraComponent->GetWorldLocation().ToString()));
GEngine->AddOnScreenDebugMessage(-1, 15.0f, FColor::Yellow, FString::Printf(TEXT("Camera Rotation: %s"), *CameraComponent->GetWorldRotation().ToString()));
GEngine->AddOnScreenDebugMessage(-1, 15.0f, FColor::Yellow, FString::Printf(TEXT("Camera FoV: %f"), CameraComponent->FieldOfView));
}
}
在这个例子中,我们使用GEngine->AddOnScreenDebugMessage
函数在屏幕上显示摄像机的当前位置、旋转和视场。
9.1.2 在Tick中更新调试信息
为了确保调试信息每次帧都更新,我们可以在Tick
函数中调用ShowCameraDebug
函数。
// 在角色类的Tick函数中更新调试信息
void AMyCharacter::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
// 目标位置和旋转
FVector TargetLocation = DesiredCameraLocation;
FRotator TargetRotation = DesiredCameraRotation;
// 使用时间插值函数进行平滑过渡
CameraComponent->SetWorldLocation(FMath::VInterpTo(CameraComponent->GetWorldLocation(), TargetLocation, DeltaTime, 10.0f));
CameraComponent->SetWorldRotation(FMath::RInterpTo(CameraComponent->GetWorldRotation(), TargetRotation, DeltaTime, 10.0f));
// 动态调整FoV
float TargetFoV = DesiredFoV;
float CurrentFoV = CameraComponent->FieldOfView;
CameraComponent->SetFieldOfView(FMath::FInterpTo(CurrentFoV, TargetFoV, DeltaTime, 2.0f));
// 检查摄像机碰撞
CheckCameraCollision();
// 更新调试信息
ShowCameraDebug();
}
在这个例子中,我们在Tick
函数中调用了ShowCameraDebug
函数,以确保摄像机的调试信息在每一帧中都得到更新。
10. 摄像机优化
10.1 优化摄像机性能
摄像机系统在运行时可能会对性能产生影响,特别是在复杂场景中。以下是一些优化摄像机性能的最佳实践和技巧。
10.1.1 减少不必要的计算
在Tick
函数中,只进行必要的摄像机计算。例如,如果摄像机的位置和旋转没有变化,不需要每次都进行插值计算。
// 在角色类中定义优化的Tick函数
void AMyCharacter::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
if (CameraComponent->GetWorldLocation() != DesiredCameraLocation)
{
CameraComponent->SetWorldLocation(FMath::VInterpTo(CameraComponent->GetWorldLocation(), DesiredCameraLocation, DeltaTime, 10.0f));
}
if (CameraComponent->GetWorldRotation() != DesiredCameraRotation)
{
CameraComponent->SetWorldRotation(FMath::RInterpTo(CameraComponent->GetWorldRotation(), DesiredCameraRotation, DeltaTime, 10.0f));
}
float CurrentFoV = CameraComponent->FieldOfView;
if (!FMath::IsNearlyEqual(CurrentFoV, DesiredFoV, 0.01f))
{
CameraComponent->SetFieldOfView(FMath::FInterpTo(CurrentFoV, DesiredFoV, DeltaTime, 2.0f));
}
CheckCameraCollision();
ShowCameraDebug();
}
在这个例子中,我们通过检查摄像机的位置、旋转和FoV是否接近目标值来减少不必要的计算。
10.1.2 使用摄像机裁剪
摄像机裁剪可以减少不必要的渲染计算,提高性能。我们可以在摄像机组件中设置裁剪平面。
// 在角色类的构造函数中设置摄像机裁剪
AMyCharacter::AMyCharacter()
{
// 创建摄像机组件
CameraComponent = CreateDefaultSubobject<UCameraComponent>(TEXT("CameraComponent"));
CameraComponent->SetupAttachment(SpringArmComponent);
// 设置摄像机裁剪平面
CameraComponent->SetNearClippingPlane(10.0f);
CameraComponent->SetFarClippingPlane(10000.0f);
}
在这个例子中,我们设置了摄像机的近裁剪面和远裁剪面,以减少不必要的渲染计算。
10.2 优化摄像机视觉效果
除了性能优化,我们还可以优化摄像机的视觉效果,以提升游戏的整体体验。
10.2.1 使用自定义纹理
在某些场景中,使用自定义纹理可以增强摄像机的视觉效果。例如,我们可以为摄像机添加一个镜头光晕或景深效果。
// 在角色类中定义添加自定义纹理的函数
void AMyCharacter::AddCustomTexture()
{
if (CameraComponent)
{
UPostProcessComponent* PostProcessComponent = NewObject<UPostProcessComponent>(this);
PostProcessComponent->SetupAttachment(CameraComponent);
// 添加镜头光晕效果
FPostProcessSettings Settings;
Settings.bOverride_LensFlare = true;
Settings.LensFlareIntensity = 1.0f;
Settings.LensFlareTint = FLinearColor(1.0f, 1.0f, 0.0f, 1.0f);
PostProcessComponent->Settings = Settings;
// 添加景深效果
Settings.bOverride_DepthOfField = true;
Settings.DepthOfFieldFocalDistance = 1000.0f;
Settings.DepthOfFieldFstop = 1.0f;
PostProcessComponent->Settings = Settings;
}
}
在这个例子中,我们为摄像机添加了一个镜头光晕和景深效果,以增强视觉效果。
11. 摄像机高级技巧
11.1 摄像机跟随路径
在某些游戏中,摄像机可能需要沿着预设的路径移动。我们可以使用Spline Component
来实现这一点。
11.1.1 创建Spline Component
首先,我们需要在角色蓝图中创建一个Spline Component
,并定义路径点。
// 在角色类的构造函数中创建Spline Component
AMyCharacter::AMyCharacter()
{
// 创建Spline Component
SplineComponent = CreateDefaultSubobject<USplineComponent>(TEXT("SplineComponent"));
SplineComponent->SetupAttachment(RootComponent);
// 添加路径点
SplineComponent->AddSplinePoint(FVector(0.0f, 0.0f, 0.0f), ESplineCoordinateSpace::World);
SplineComponent->AddSplinePoint(FVector(1000.0f, 0.0f, 0.0f), ESplineCoordinateSpace::World);
SplineComponent->AddSplinePoint(FVector(1000.0f, 1000.0f, 0.0f), ESplineCoordinateSpace::World);
}
11.1.2 沿路径移动摄像机
在Tick
函数中,我们可以使用Spline Component
的方法来沿路径移动摄像机。
// 在角色类中定义沿路径移动摄像机的函数
void AMyCharacter::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
// 沿路径移动摄像机
float PathLength = SplineComponent->GetSplineLength();
float CurrentTime = (FMath::Fmod(GetWorld()->GetTimeSeconds(), PathLength) / PathLength) * 100.0f;
FVector CameraLocation = SplineComponent->GetLocationAtDistanceAlongSpline(CurrentTime, ESplineCoordinateSpace::World);
FRotator CameraRotation = SplineComponent->GetRotationAtDistanceAlongSpline(CurrentTime, ESplineCoordinateSpace::World);
CameraComponent->SetWorldLocation(CameraLocation);
CameraComponent->SetWorldRotation(CameraRotation);
// 其他摄像机逻辑
if (CameraComponent->GetWorldLocation() != DesiredCameraLocation)
{
CameraComponent->SetWorldLocation(FMath::VInterpTo(CameraComponent->GetWorldLocation(), DesiredCameraLocation, DeltaTime, 10.0f));
}
if (CameraComponent->GetWorldRotation() != DesiredCameraRotation)
{
CameraComponent->SetWorldRotation(FMath::RInterpTo(CameraComponent->GetWorldRotation(), DesiredCameraRotation, DeltaTime, 10.0f));
}
float CurrentFoV = CameraComponent->FieldOfView;
if (!FMath::IsNearlyEqual(CurrentFoV, DesiredFoV, 0.01f))
{
CameraComponent->SetFieldOfView(FMath::FInterpTo(CurrentFoV, DesiredFoV, DeltaTime, 2.0f));
}
CheckCameraCollision();
ShowCameraDebug();
}
在这个例子中,我们使用Spline Component
的GetLocationAtDistanceAlongSpline
和GetRotationAtDistanceAlongSpline
方法来沿路径移动和旋转摄像机。
11.2 摄像机模糊效果
模糊效果可以用于模拟焦外模糊或运动模糊,增强游戏的视觉体验。我们可以使用Post Process Volume
中的模糊效果设置来实现这一点。
11.2.1 设置模糊效果
在Post Process Volume
的详细信息面板中,我们可以设置Motion Blur
和Depth of Field
效果。
-
在场景中创建一个
Post Process Volume
。 -
在
Post Process Volume
的详细信息面板中,添加一个Post Process Blend Weight
参数,并设置其权重。 -
设置
Motion Blur
和Depth of Field
效果的参数。
11.2.2 动态调整模糊效果
在角色类中,我们可以定义一个函数来动态调整模糊效果的参数。
// 在角色类中定义动态调整模糊效果的函数
void AMyCharacter::AdjustBlurEffect(float NewMotionBlurAmount, float NewDepthOfFieldFocalDistance)
{
if (PostProcessVolume)
{
PostProcessVolume->Settings.MotionBlurAmount = NewMotionBlurAmount;
PostProcessVolume->Settings.DepthOfFieldFocalDistance = NewDepthOfFieldFocalDistance;
}
}
11.2.3 在游戏逻辑中应用模糊效果
我们可以在游戏逻辑中根据需要调用AdjustBlurEffect
函数,例如在角色快速移动时增加运动模糊效果。
// 在角色类中定义快速移动函数
void AMyCharacter::MoveFast(float Value)
{
if (Value != 0.0f)
{
// 处理快速移动逻辑
AddMovementInput(GetActorForwardVector(), Value);
// 增加运动模糊效果
AdjustBlurEffect(0.8f, 1000.0f);
}
}
// 在角色类中定义恢复模糊效果的函数
void AMyCharacter::RecoverBlurEffect()
{
// 恢复模糊效果
AdjustBlurEffect(0.0f, 1000.0f);
}
// 在角色类中定义输入处理函数
void AMyCharacter::SetupPlayerInputComponent(class UInputComponent* PlayerInputComponent)
{
Super::SetupPlayerInputComponent(PlayerInputComponent);
PlayerInputComponent->BindAxis("MoveForward", this, &AMyCharacter::MoveFast);
PlayerInputComponent->BindAxis("LookUp", this, &AMyCharacter::LookUp);
PlayerInputComponent->BindAxis("Turn", this, &AMyCharacter::Turn);
// 设置摄像机旋转限制
SetCameraRotationLimits(-45.0f, 45.0f);
}
在这个例子中,当角色快速移动时,我们增加了运动模糊效果,并在角色停止移动时恢复到正常状态。
12. 总结
通过以上最佳实践和技巧,开发者可以在Unreal Engine中实现更加流畅、自然且功能丰富的摄像机系统。摄像机平滑过渡、跟随、抖动、FoV调整、碰撞检测、切换、视角限制和视觉效果优化都是提升游戏体验的重要方面。希望这些内容能够帮助你在虚拟摄像机开发中取得更好的成果。
如果你有任何其他问题或需要进一步的帮助,请随时联系我。祝你开发顺利!