A Knight’s Journey
Time Limit: 1000MS Memory Limit: 65536K
Total Submissions: 60600 Accepted: 20722
Description
Background
The knight is getting bored of seeing the same black and white squares again and again and has decided to make a journey
around the world. Whenever a knight moves, it is two squares in one direction and one square perpendicular to this. The world of a knight is the chessboard he is living on. Our knight lives on a chessboard that has a smaller area than a regular 8 * 8 board, but it is still rectangular. Can you help this adventurous knight to make travel plans?
Problem
Find a path such that the knight visits every square once. The knight can start and end on any square of the board.
Input
The input begins with a positive integer n in the first line. The following lines contain n test cases. Each test case consists of a single line with two positive integers p and q, such that 1 <= p * q <= 26. This represents a p * q chessboard, where p describes how many different square numbers 1, . . . , p exist, q describes how many different square letters exist. These are the first q letters of the Latin alphabet: A, . . .
Output
The output for every scenario begins with a line containing “Scenario #i:”, where i is the number of the scenario starting at 1. Then print a single line containing the lexicographically first path that visits all squares of the chessboard with knight moves followed by an empty line. The path should be given on a single line by concatenating the names of the visited squares. Each square name consists of a capital letter followed by a number.
If no such path exist, you should output impossible on a single line.
Sample Input
3
1 1
2 3
4 3
Sample Output
Scenario #1:
A1
Scenario #2:
impossible
Scenario #3:
A1B3C1A2B4C2A3B1C3A4B2C4
描述
背景
骑士无聊一次又一次看到相同的黑白方块,因此决定
环游世界。每当骑士移动时,它在一个方向上是两个正方形,而垂直于该方向的正方形是一个正方形。骑士的世界就是他赖以生存的棋盘。我们的骑士生活在棋盘上,棋盘的面积比普通的8 * 8棋盘小,但它仍然是矩形的。您可以帮助这个冒险的骑士制定旅行计划吗?
问题
找到一条路径,使骑士可以拜访每个广场一次。骑士可以在棋盘的任何正方形上开始和结束。
输入值
输入在第一行中以正整数n开头。以下各行包含n个测试用例。每个测试用例都由一条带有两个正整数p和q的单行组成,因此1 <= p * q <=26。这表示ap * q棋盘,其中p描述了多少个不同的平方1。。。,p存在,q描述存在多少个不同的正方形字母。这些是拉丁字母的前q个字母:A 、。。。
输出量
每个方案的输出都以包含“方案#i:”的行开头,其中i是从1开始的方案编号。然后打印一行,按字典顺序,第一个路径通过骑士移动来访问棋盘的所有正方形用空行。应通过合并访问的正方形的名称在一行上给出路径。每个正方形名称均由大写字母后跟数字组成。
如果不存在这样的路径,则不应在单行上输出不可能。
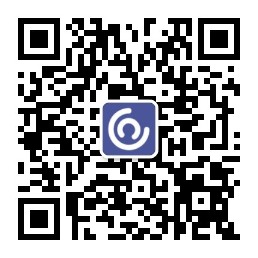
JAVA代码
import java.util.LinkedList;
import java.util.Queue;
import java.util.Scanner;
/*
* poj 2488 骑士周游
*/
public class Main {
static int fir[][]={
{
-1,-2},{
1,-2},{
-2,-1},{
2,-1},{
-2,1},{
2,1},{
-1,2},{
1,2}};
static int n,m;
static int flat[][];
static int op;
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int id = 1;
int k = sc.nextInt();
for(int i=0;i<k;i++){
op=1;
n = sc.nextInt();
m = sc.nextInt();
flat = new int[n+1][m+1];
boolean bool = false;
Horse h = new Horse(1, 1, 1, "A1");
System.out.println("Scenario #"+id+++":");
DFS(h,flat);
if(op==1) {
System.out.println("impossible");
}
if(i<k-1) {
System.out.println();
}
}
}
public static void DFS(Horse h,int[][]flat) {
if(h.step==n*m&&op==1) {
System.out.println(h.path);
op=0;
return;
}else {
flat[h.x][h.y] = 1;
for(int l=0;l<8;l++) {
int x = h.x+fir[l][0];
int y = h.y+fir[l][1];
if(x>=1&&x<=n&&y>=1&&y<=m&&flat[x][y]==0) {
Horse hor = new Horse(x, y, h.step+1, h.path+(char)(y+'A'-1)+x);
DFS(hor, flat);
}
}
flat[h.x][h.y] = 0;
}
}
}
class Horse{
int x,y,step;
String path;
public Horse(int x, int y, int step, String path) {
super();
this.x = x;
this.y = y;
this.step = step;
this.path = path;
}
}
C++代码:
#include <iostream>
#include <cstring>
using namespace std;
/* run this program using the console pauser or add your own getch, system("pause") or input loop */
int k,n,m;
int flat[8][8];
int op; //步数 ,标记
int fir[8][2]={
{
-1,-2},{
1,-2},{
-2,-1},{
2,-1},{
-2,1},{
2,1},{
-1,2},{
1,2}};
void DFS(int x,int y,int step,int path[30][2]){
path[step][0] = x;
path[step][1] = y;
if(step==n*m&&op==1){
op = 0;
for(int i=1;i<=n*m;i++){
cout<<(char)(path[i][1]-1+'A')<<path[i][0];
}
cout<<endl;
return;
}else{
flat[x][y] = 1;
for(int i=0;i<8;i++){
int xx = x+fir[i][0];
int yy = y+fir[i][1];
if(xx>=1&&xx<=n&&yy>=1&&yy<=m&&flat[xx][yy]==0){
DFS(xx,yy,step+1,path);
}
}
flat[x][y] = 0;
}
}
int main(int argc, char *argv[]) {
int id = 1;
cin>>k;
while(k--){
cin>>n>>m;
op = 1;
memset(flat,0,sizeof(flat));
int path[30][2];
cout<<"Scenario #"<<id++<<":"<<endl;
DFS(1,1,1,path);
if(op){
cout<<"impossible"<<endl;
}
cout<<endl;
}
return 0;
}