描述
给定一个由整数组成二维矩阵(r*c),现在需要找出它的一个子矩阵,使得这个子矩阵内的所有元素之和最大,并把这个子矩阵称为最大子矩阵。
例子:
0 -2 -7 0
9 2 -6 2
-4 1 -4 1
-1 8 0 -2
其最大子矩阵为:
9 2
-4 1
-1 8
其元素总和为15。
输入
第一行输入一个整数n(0<n<=100),表示有n组测试数据;
每组测试数据:
第一行有两个的整数r,c(0<r,c<=100),r、c分别代表矩阵的行和列;
随后有r行,每行有c个整数;
输出
输出矩阵的最大子矩阵的元素之和。
样例输入
1
4 4
0 -2 -7 0
9 2 -6 2
-4 1 -4 1
-1 8 0 -2
样例输出
15
思路:乍一看该题好像很难,无从下手,更别说理清初始状态与末状态的关系。但实际上该题只需要小小的转化一下便成为了经典DP问题。(需要大胆的联想与猜测,想错了又不扣钱)
联想:求最大子矩阵和,联想到经典DP中的最大子序列和。但矩阵是二维的,如何将二维问题转化为一维?
扫描二维码关注公众号,回复:
2843510 查看本文章
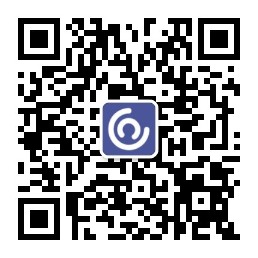
转化:获取最大的过程一定是max一下,那就是每个矩阵都可以表示为一个数(它的和),如何求得它的和?我们需要考虑矩阵的取法,以某一行/列为基准,有序的取小矩形。那么我们就可以把矩阵上下边界固定,左右移动获得子矩阵,再移动边界获得其他矩阵,那这个问题就转化的差不多了,枚举行数i.j,把每列数相加,每个小矩形即为每列和累加,求这个区间子矩阵最大就成为了一个一维数组求最大子序列和问题,更新到最后输出即可。
代码如下:
#include<iostream>
#include<algorithm>
#include<stdio.h>
#include<string.h>
#include<string>
#include<set>
#include<map>
#include<list>
#include<queue>
#include<vector>
#include<cmath>
#include<stdlib.h>
#include<ext/rope>
#define per(i,a,b) for(int i=a;i<=b;i++)
#define inf 0x3f3f3f3f
#define pi acos(-1.0)
using namespace std;
using namespace __gnu_cxx;
#define N 1001
template <class T>
inline bool scan_d(T &ret)
{
char c; int sgn;
if (c = getchar(), c == EOF) return 0; //EOF
while (c != '-' && (c < '0' || c > '9')) c = getchar();
sgn = (c == '-') ? -1 : 1;
ret = (c == '-') ? 0 : (c - '0');
while (c = getchar(), c >= '0' && c <= '9') ret = ret * 10 + (c - '0');
ret *= sgn;
return 1;
}
int p[200][200]={0},maxn,n,m;
void what(int x)
{
int t=0;
per(i,1,m)
{
if(t>0) t+=p[x][i];
else t=p[x][i];
maxn=max(t,maxn);
}
}
int main()
{
int t;
scanf("%d",&t);
while(t--)
{
maxn=-inf;
memset(p,0,sizeof(p));
scanf("%d%d",&n,&m);
per(i,1,n)
{
per(j,1,m)
{
scan_d(p[i][j]);
}
}
per(i,1,n)
{
what(i);
per(j,i+1,n)
{
per(k,1,m)
{
p[i][k]+=p[j][k];
}
what(i);
}
}
printf("%d\n",maxn);
}
return 0;
}