第一题:
网友年龄
某君新认识一网友。
当问及年龄时,他的网友说:
“我的年龄是个2位数,我比儿子大27岁,
如果把我的年龄的两位数字交换位置,刚好就是我儿子的年龄”
请你计算:网友的年龄一共有多少种可能情况?
提示:30岁就是其中一种可能哦.
答案:7种
#include <iostream>
#include <cstring>
#include <cstdio>
#include <algorithm>
#include <cmath>
using namespace std;
const int mod = 1e9 + 7;
typedef long long LL;
int n, m;
int main()
{
for(int i = 27; i <= 99; i ++ )
{
if(i - ((i % 10) * 10 + i / 10) == 27) printf("%d\n", i);
}
return 0;
}
第二题:
生日蜡烛
某君从某年开始每年都举办一次生日party,并且每次都要吹熄与年龄相同根数的蜡烛。
现在算起来,他一共吹熄了236根蜡烛。
请问,他从多少岁开始过生日party的?
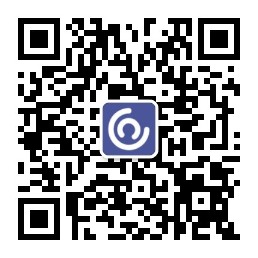
请填写他开始过生日party的年龄数。
答案为26。
第三题:
#include <cstdio>
#include <queue>
#include <map>
#include <cmath>
#include <vector>
#include <cstring>
#include <iostream>
#include <stack>
#include <algorithm>
using namespace std;
const int r = 3, c = 4;
int mapp[10][10];
int numv[15];
int cou;
int dir[4][2] = {
0, -1, -1, -1, -1, 0, -1, 1 };
bool check(int x, int y, int n) {
for (int i = 0; i < 4; i++) {
int nx = x + dir[i][0];
int ny = y + dir[i][1];
if (nx >= 0 && nx < r && ny >= 0 && ny < c) {
//相邻的点有相邻的数字
//还没有填过数字的格子-10 肯定不会等于当前格子0-9 +1或-1
if (mapp[nx][ny] == n - 1 || mapp[nx][ny] == n + 1) {
return false;
}
}
}
return true;
}
void dfs(int dep, int pos) {
//(2,3)为缺口,结束
if (dep == 2 && pos == 3) {
cou++;
return;
}
if (pos >= c) {
dfs(dep + 1, 0);
}
else {
for (int i = 0; i <= 9; i++) {
//这个数i没用过,并且没有越出格子
if (!numv[i] && check(dep, pos, i)) {
numv[i] = true;
mapp[dep][pos] = i;
dfs(dep, pos + 1);
mapp[dep][pos] = -10;
numv[i] = false;
}
}
}
}
int main()
{
//初始化为一个比较大的负数,那么在
for (int i = 0; i <= 5; i++) {
for (int j = 0; j <= 5; j++) {
mapp[i][j] = -10;
}
}
memset(numv, false, sizeof(numv));
cou = 0;
dfs(0, 1); //(0,0)为缺口,所以从(0,1)开始
cout << cou << endl; //1580
return 0;
}
第四题:
快速排序
排序在各种场合经常被用到。
快速排序是十分常用的高效率的算法。
其思想是:先选一个“标尺”,
用它把整个队列过一遍筛子,
以保证:其左边的元素都不大于它,其右边的元素都不小于它。
这样,排序问题就被分割为两个子区间。
再分别对子区间排序就可以了。
下面的代码是一种实现,请分析并填写划线部分缺少的代码
#include <stdio.h>
void swap(int a[], int i, int j)
{
int t = a[i];
a[i] = a[j];
a[j] = t;
}
int partition(int a[], int p, int r)
{
int i = p;
int j = r + 1;
int x = a[p];
while(1){
while(i<r && a[++i]<x);
while(a[--j]>x);
if(i>=j) break;
swap(a,i,j);
}
______________________;
//swap(a, p, j);
return j;
}
void quicksort(int a[], int p, int r)
{
if(p<r){
int q = partition(a,p,r);
quicksort(a,p,q-1);
quicksort(a,q+1,r);
}
}
int main()
{
int i;
int a[] = {
5,13,6,24,2,8,19,27,6,12,1,17};
int N = 12;
quicksort(a, 0, N-1);
for(i=0; i<N; i++) printf("%d ", a[i]);
printf("\n");
return 0;
}
第五题:
消除尾一
下面的代码把一个整数的二进制表示的最右边的连续的1全部变成0
如果最后一位是0,则原数字保持不变。
如果采用代码中的测试数据,应该输出:
00000000000000000000000001100111 00000000000000000000000001100000
00000000000000000000000000001100 00000000000000000000000000001100
请仔细阅读程序,填写划线部分缺少的代码。
#include <stdio.h>
void f(int x)
{
int i;
for(i=0; i<32; i++) printf("%d", (x>>(31-i))&1);
printf(" ");
x = _______________________;//x & x + 1;
for(i=0; i<32; i++) printf("%d", (x>>(31-i))&1);
printf("\n");
}
int main()
{
f(103);
f(12);
return 0;
}
第六题:
寒假作业
现在小学的数学题目也不是那么好玩的。
看看这个寒假作业:
□ + □ = □
□ - □ = □
□ × □ = □
□ ÷ □ = □
(如果显示不出来,可以参见【图1.jpg】)
每个方块代表1~13中的某一个数字,但不能重复。
比如:
6 + 7 = 13
9 - 8 = 1
3 * 4 = 12
10 / 2 = 5
以及:
7 + 6 = 13
9 - 8 = 1
3 * 4 = 12
10 / 2 = 5
就算两种解法。(加法,乘法交换律后算不同的方案)
你一共找到了多少种方案?
#include <iostream>
#include <cstring>
using namespace std;
int a[15];
bool st[15];
int res = 0;
void dfs(int x)
{
if(x >= 4 && a[1] + a[2] != a[3]) return ;
if(x >= 7 && a[4] - a[5] != a[6]) return ;
if(x >= 10 && a[7] * a[8] != a[9]) return ;
if(x >= 13 && a[12] * a[11] == a[10])
{
res ++ ;
return ;
}
for(int i = 1; i <= 13; i ++ )
{
if(!st[i])
{
a[x] = i;
st[i] = 1;
dfs(x + 1);
st[i] = 0;
}
}
}
int main()
{
dfs(1);
cout << res << endl;
return 0;
}
第七题:
剪邮票
如【图1.jpg】, 有12张连在一起的12生肖的邮票。
现在你要从中剪下5张来,要求必须是连着的。
(仅仅连接一个角不算相连)
比如,【图2.jpg】,【图3.jpg】中,粉红色所示部分就是合格的剪取。
请你计算,一共有多少种不同的剪取方法。
请填写表示方案数目的整数。
#include <iostream>
#include <cstring>
#include <algorithm>
using namespace std;
int dir[4] = {
1, -1, 5, -5};
int temp[12] = {
1,2,3,4,6,7,8,9,11,12,13,14};
int temp2[5];
int vis[5];
int ans = 0;
void dfs(int u)
{
for(int i = 0; i < 4; i ++ )
{
int t = temp2[u] + dir[i];
if(t >= 1 && t <= 14)
{
for(int j = 0; j < 5; j ++ )
if(t == temp2[j] && !vis[j])
{
vis[j] = 1;
dfs(j);
}
}
}
}
int main()
{
for(int a = 0; a < 12; a ++ )
for(int b = a + 1; b < 12; b ++ )
for(int c = b + 1; c < 12; c ++ )
for(int d = c + 1; d < 12; d ++ )
for(int e = d + 1; e < 12; e ++ )
{
temp2[0] = temp[a];
temp2[1] = temp[b];
temp2[2] = temp[c];
temp2[3] = temp[d];
temp2[4] = temp[e];
memset(vis, 0, sizeof vis);
vis[0] = 1;
dfs(0);
bool flag = true;
for(int i = 0; i < 5; i ++ )
if(vis[i] == 0)
flag = 0;
if(flag) ans ++ ;
}
printf("%d\n", ans);
return 0;
}
第八题:
比如:
5 = 0^2 + 0^2 + 1^2 + 2^2
7 = 1^2 + 1^2 + 1^2 + 2^2
(^符号表示乘方的意思)
对于一个给定的正整数,可能存在多种平方和的表示法。
要求你对4个数排序:
0 <= a <= b <= c <= d
并对所有的可能表示法按 a,b,c,d 为联合主键升序排列,最后输出第一个表示法
程序输入为一个正整数N (N<5000000)
要求输出4个非负整数,按从小到大排序,中间用空格分开
例如,输入:
5
则程序应该输出:
0 0 1 2
再例如,输入:
12
则程序应该输出:
0 2 2 2
再例如,输入:
773535
则程序应该输出:
1 1 267 838
#include <stdio.h>
#include <math.h>
int main()
{
int a, b, c, n, flag = 0;
double maxN, d;
scanf("%d", &n);
maxN = sqrt(n);
for(a = 0; a <= maxN; a ++){
for(b = a; b <= maxN; b ++){
for(c = b; c <= maxN; c ++){
d = sqrt(n - a*a - b*b - c*c);
if(d == (int)d){
printf("%d %d %d %d\n", a, b, c, (int)d);
return 0;
}
}
}
}
}
第九题:
密码脱落
X星球的考古学家发现了一批古代留下来的密码。
这些密码是由A、B、C、D 四种植物的种子串成的序列。
仔细分析发现,这些密码串当初应该是前后对称的(也就是我们说的镜像串)。
由于年代久远,其中许多种子脱落了,因而可能会失去镜像的特征。
你的任务是:
给定一个现在看到的密码串,计算一下从当初的状态,它要至少脱落多少个种子,才可能会变成现在的样子。
输入一行,表示现在看到的密码串(长度不大于1000)
要求输出一个正整数,表示至少脱落了多少个种子。
例如,输入:
ABCBA
则程序应该输出:
0
再例如,输入:
ABDCDCBABC
则程序应该输出:
3
资源约定:
峰值内存消耗 < 256M
CPU消耗 < 1000ms
#include <stdio.h>
#include <string.h>
char s[1000];
int min = 0, num = 0;
void fcode(int left, int right, int num)
{
if (left >= right)
{
min = min < num ? min : num;
}
else
{
if (s[left] == s[right])
{
fcode(left + 1, right - 1, num);
}
else
{
fcode(left + 1, right, num + 1);
fcode(left, right - 1, num + 1);
}
}
return ;
}
int main()
{
scanf("%s", s);
min = (int)strlen(s);
//处理密码
fcode(0, min - 1, 0);
printf("%d\n", min);
return 0;
}
第十题:
最大比例
X星球的某个大奖赛设了M级奖励。每个级别的奖金是一个正整数。
并且,相邻的两个级别间的比例是个固定值。
也就是说:所有级别的奖金数构成了一个等比数列。比如:
16,24,36,54
其等比值为:3/2
现在,我们随机调查了一些获奖者的奖金数。
请你据此推算可能的最大的等比值。
输入格式:
第一行为数字 N (0<N<100),表示接下的一行包含N个正整数
第二行N个正整数Xi(Xi<1 000 000 000 000),用空格分开。每个整数表示调查到的某人的奖金数额
要求输出:
一个形如A/B的分数,要求A、B互质。表示可能的最大比例系数
测试数据保证了输入格式正确,并且最大比例是存在的。
例如,输入:
3
1250 200 32
程序应该输出:
25/4
再例如,输入:
4
3125 32 32 200
程序应该输出:
5/2
再例如,输入:
3
549755813888 524288 2
程序应该输出:
4/1
#include<cstdio>
#include<cstring>
#include<iostream>
#include<algorithm>
#include<string>
using namespace std;
long long gcd(long long a,long long b)//求最大公约数,怕数值越出,所以用long long
{
long long t;
while(t=a%b)
{
a=b;
b=t;
}
return b;
}
int main()
{
long long a[100]={
0},p1[100]={
0},p2[100]={
0},t,g,t1,t2;
int n,i,j,k;
scanf("%d",&n);
for(i=0;i<n;i++)
scanf("%lld",&a[i]);//处理输入
sort(a,a+n); //排序
j=1;
for(i=1;i<n;i++) //去重
{
if(a[i]!=a[i-1])
a[j++]=a[i];
}//这里也可以考虑用set集合去重
n=j;
if(n==1) //只有一个数
printf("1/1\n");
else if(n==2) //有两个数
{
g=gcd(a[n-1],a[n-2]);
printf("%lld/%lld\n",a[n-1]/g,a[n-2]/g);
}
else if(n>2)
{
k=0;
for(i=1;i<n;i++) //分别求出后一项和前一项的比值
{
g=gcd(a[i],a[i-1]);
p1[k]=a[i]/g;
p2[k]=a[i-1]/g;
k++;
}
for(i=0;i<k;i++) //找出最小公比
{
for(j=i+1;j<k;j++)
{
if(p1[i]*p2[j]>p1[j]*p2[i]) //p1[i]/p2[i]比p1[j]/p2[j]大 p1[i]/p2[i]除p1[j]/p2[j]
{
t1=p1[i]/p1[j];
t2=p2[i]/p2[j];
}
else if(p1[i]*p2[j]<p1[j]*p2[i])
{
t1=p1[j]/p1[i];
t2=p2[j]/p2[i];
}
else if(p1[i]*p2[j]==p1[j]*p2[i])
{
t1=p1[i];
t2=p2[i];
}
}
}
g=gcd(t1,t2);
printf("%lld/%lld\n",t1/g,t2/g);
}
return 0;
}